编写python程序,要求采用迭代算法获取阈值,并进行图像阈值分割
时间: 2024-12-12 09:31:54 浏览: 5
在Python中,我们可以使用OpenCV库来进行图像处理,特别是图像的阈值分割。这个过程通常涉及到找到一个合适的阈值,使得图像被分为前景和背景两个部分。下面是一个简单的示例,使用了Otsu's方法(一种常见的自动阈值确定方法)作为迭代算法:
```python
import cv2
import numpy as np
def get_threshold(image):
# 将图像转换为灰度
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 计算Otsu's阈值
_, threshold = cv2.threshold(gray_image, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
return threshold
def iterative_thresholding(image, low=0, high=256, step=1):
best_threshold = None
best_energy = 0
for threshold in range(low, high, step):
binary = (gray_image > threshold).astype('uint8')
energy = np.sum(binary * binary) # 能量计算,类似于方差
if energy > best_energy:
best_energy = energy
best_threshold = threshold
return best_threshold
# 使用图片路径
image_path = 'your_image.jpg'
image = cv2.imread(image_path)
# 获取初始阈值
initial_threshold = get_threshold(image)
# 迭代寻找最佳阈值
optimal_threshold = iterative_thresholding(image, initial_threshold - 20, initial_threshold + 20, 1)
# 对原始图像应用优化的阈值
binary_image = (gray_image > optimal_threshold).astype('uint8')
# 显示原图和二值化后的结果
cv2.imshow('Original Image', image)
cv2.imshow('Thresholded Image', binary_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
阅读全文
相关推荐
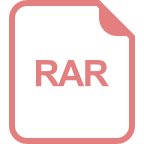
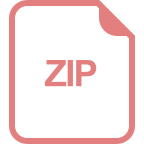
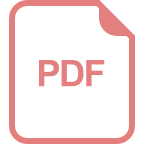
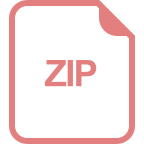
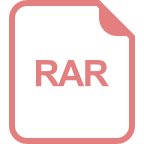
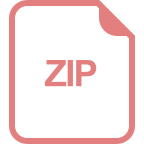
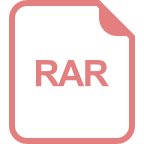
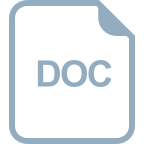
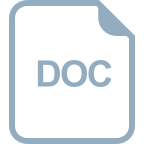
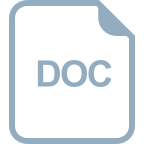
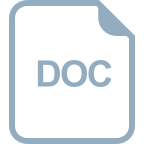
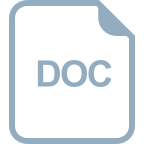
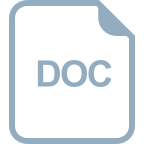
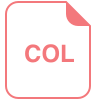
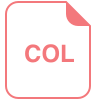
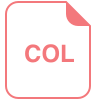
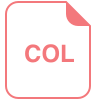

