winform 圆角窗体
时间: 2023-11-15 21:07:20 浏览: 92
Winform圆角窗体可以通过自定义类和继承实现。具体步骤如下:
1. 创建项目,添加自定义类:MyWin32.cs和PerPixelAlphaBlend.cs;圆角窗体RoundedForm ;继承RoundedForm的窗体Form1。
2. 在MyWin32.cs中添加以下代码:
```csharp
using System;
using System.Runtime.InteropServices;
namespace Win32
{
public static class MyWin32
{
[DllImport("user32.dll")]
public static extern IntPtr GetDC(IntPtr hWnd);
[DllImport("user32.dll")]
public static extern int ReleaseDC(IntPtr hWnd, IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern IntPtr CreateCompatibleDC(IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern int DeleteDC(IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern IntPtr SelectObject(IntPtr hDC, IntPtr hObject);
[DllImport("gdi32.dll")]
public static extern int DeleteObject(IntPtr hObject);
[DllImport("user32.dll")]
public static extern int GetWindowRect(IntPtr hWnd, out RECT lpRect);
[StructLayout(LayoutKind.Sequential)]
public struct RECT
{
public int Left;
public int Top;
public int Right;
public int Bottom;
}
}
}
```
3. 在PerPixelAlphaBlend.cs中添加以下代码:
```csharp
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.Runtime.InteropServices;
namespace Win32
{
public static class PerPixelAlphaBlend
{
[DllImport("user32.dll")]
public static extern IntPtr GetDC(IntPtr hWnd);
[DllImport("user32.dll")]
public static extern int ReleaseDC(IntPtr hWnd, IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern IntPtr CreateCompatibleDC(IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern int DeleteDC(IntPtr hDC);
[DllImport("gdi32.dll")]
public static extern IntPtr SelectObject(IntPtr hDC, IntPtr hObject);
[DllImport("gdi32.dll")]
public static extern int DeleteObject(IntPtr hObject);
[DllImport("msimg32.dll", EntryPoint = "AlphaBlend")]
public static extern bool AlphaBlend(IntPtr hdcDest, int nXOriginDest, int nYOriginDest, int nWidthDest, int nHeightDest, IntPtr hdcSrc, int nXOriginSrc, int nYOriginSrc, int nWidthSrc, int nHeightSrc, BLENDFUNCTION blendFunction);
[StructLayout(LayoutKind.Sequential)]
public struct BLENDFUNCTION
{
public byte BlendOp;
public byte BlendFlags;
public byte SourceConstantAlpha;
public byte AlphaFormat;
}
public static void SetBitmap(Bitmap bitmap, Control control)
{
SetBitmap(bitmap, control, 255);
}
public static void SetBitmap(Bitmap bitmap, Control control, byte opacity)
{
if (control == null)
{
throw new ArgumentNullException("control");
}
IntPtr screenDc = GetDC(IntPtr.Zero);
IntPtr memDc = CreateCompatibleDC(screenDc);
IntPtr hBitmap = IntPtr.Zero;
IntPtr oldBitmap = IntPtr.Zero;
try
{
hBitmap = bitmap.GetHbitmap(Color.FromArgb(0));
oldBitmap = SelectObject(memDc, hBitmap);
Size size = new Size(bitmap.Width, bitmap.Height);
Point pointSource = new Point(0, 0);
Point topPos = new Point(control.Left, control.Top);
Win32.MyWin32.BLENDFUNCTION blend = new Win32.MyWin32.BLENDFUNCTION();
blend.BlendOp = 0;
blend.BlendFlags = 0;
blend.SourceConstantAlpha = opacity;
blend.AlphaFormat = 1;
Win32.MyWin32.RECT sourceRect = new Win32.MyWin32.RECT()
{
Left = pointSource.X,
Top = pointSource.Y,
Right = pointSource.X + size.Width,
Bottom = pointSource.Y + size.Height
};
Win32.MyWin32.RECT destRect = new Win32.MyWin32.RECT()
{
Left = topPos.X,
Top = topPos.Y,
Right = topPos.X + size.Width,
Bottom = topPos.Y + size.Height
};
IntPtr screenDc2 = GetDC(IntPtr.Zero);
AlphaBlend(screenDc2, destRect.Left, destRect.Top, destRect.Right - destRect.Left, destRect.Bottom - destRect.Top, memDc, sourceRect.Left, sourceRect.Top, sourceRect.Right - sourceRect.Left, sourceRect.Bottom - sourceRect.Top, blend);
ReleaseDC(IntPtr.Zero, screenDc2);
}
finally
{
SelectObject(memDc, oldBitmap);
DeleteObject(hBitmap);
DeleteDC(memDc);
ReleaseDC(IntPtr.Zero, screenDc);
}
}
}
}
```
4. 在RoundedForm.cs中添加以下代码:
```csharp
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Windows.Forms;
namespace Win32
{
public class RoundedForm : Form
{
private int radius = 20;
public int Radius
{
get { return radius; }
set { radius = value; }
}
protected override void OnPaint(PaintEventArgs e)
{
GraphicsPath path = GetRoundPath(ClientRectangle, radius);
this.Region = new Region(path);
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
e.Graphics.FillPath(new SolidBrush(Color.White), path);
}
private GraphicsPath GetRoundPath(RectangleF rect, int radius)
{
float r2 = radius / 2f;
GraphicsPath path = new GraphicsPath();
path.AddArc(rect.X, rect.Y, radius, radius, 180, 90);
path.AddLine(rect.X + r2, rect.Y, rect.Width - r2, rect.Y);
path.AddArc(rect.X + rect.Width - radius, rect.Y, radius, radius, 270, 90);
path.AddLine(rect.Width, rect.Y + r2, rect.Width, rect.Height - r2);
path.AddArc(rect.X + rect.Width - radius, rect.Y + rect.Height - radius, radius, radius, 0, 90);
path.AddLine(rect.Width - r2, rect.Height, rect.X + r2, rect.Height);
path.AddArc(rect.X, rect.Y + rect.Height - radius, radius, radius, 90, 90);
path.AddLine(rect.X, rect.Height - r2, rect.X, rect.Y + r2);
path.CloseFigure();
return path;
}
}
}
```
5. 在Form1.cs中继承RoundedForm,并添加以下代码:
```csharp
public partial class Form1 : RoundedForm
{
public Form1()
{
InitializeComponent();
this.Radius = 20;
}
}
```
6. 在Form1.Designer.cs中添加以下代码:
```csharp
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(284, 261);
this.Name = "Form1";
this.Text = "Form1";
```
7. 在Form1_Load事件中添加以下代码:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
this.BackColor = Color.FromArgb(0, 0, 0, 0);
PerPixelAlphaBlend.SetBitmap(Properties.Resources.background, this);
}
```
其中,background是一个png格式的图片,用于设置窗体背景。
阅读全文
相关推荐
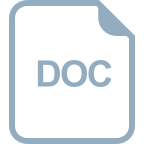
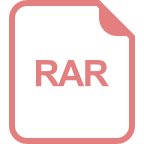
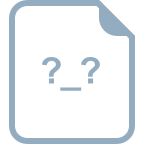
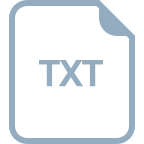

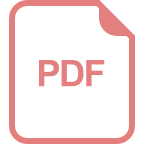
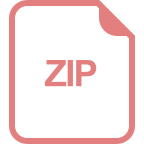
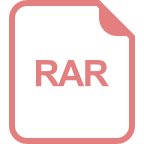
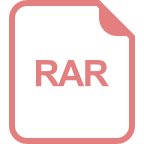
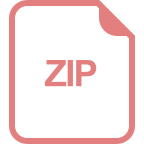
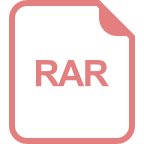
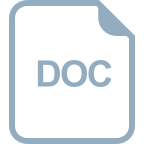


