Arduino Init
时间: 2024-08-16 20:04:42 浏览: 41
Arduino初始化(Init)通常是指在Arduino程序中设置硬件环境、加载库函数以及准备开始执行操作的阶段。当你创建一个新的Arduino项目时,初始化过程主要包括以下几个步骤:
1. **包含头文件**:首先,你需要包含Arduino的核心头文件`<Arduino.h>`,这个文件包含了基本的硬件抽象层,如数字和模拟输入输出(I/O)管理。
2. **初始化硬件**:通过调用`setup()`函数,可以配置板子上可用的硬件资源。这包括设置LED的状态、连接传感器等,并分配给它们相应的数字引脚。
3. **声明并初始化变量**:在这里,你可以定义全局变量并初始化它们,比如计数器、数据存储等。
4. **设置串口通信**:如果需要与其他设备通讯,会在`setup()`里开启Serial或其他通信端口。
5. **主循环**:大部分的代码会放在`loop()`函数中,这是一个无限循环,负责程序的主要执行逻辑。`setup()`只执行一次,而`loop()`则反复运行。
```cpp
void setup() {
// 初始化硬件
Serial.begin(9600); // 开启串口通信
// 其他初始化操作...
}
void loop() {
// 主循环代码...
}
```
相关问题
arduino上 Radio.Init
我不知道您想要什么类型的无线电,因为不同类型的无线电需要不同的库和初始化过程。以下是一些常见的无线电初始化过程:
1. NRF24L01无线电:
使用RF24库初始化NRF24L01无线电:
```
#include <SPI.h>
#include <RF24.h>
RF24 radio(9, 10); // CE, CSN pins
void setup() {
radio.begin();
radio.setChannel(108); // 设置频道为108
radio.setDataRate(RF24_2MBPS); // 设置数据速率为2Mbps
radio.setPALevel(RF24_PA_MAX); // 设置功率为最大
}
void loop() {
// 发送数据
const char text[] = "Hello World";
radio.write(&text, sizeof(text));
delay(1000);
}
```
2. HC-05蓝牙模块:
使用SoftwareSerial库初始化HC-05蓝牙模块:
```
#include <SoftwareSerial.h>
SoftwareSerial BTSerial(2, 3); // RX, TX pins
void setup() {
BTSerial.begin(9600);
}
void loop() {
// 发送数据
BTSerial.println("Hello World");
delay(1000);
}
```
3. ESP8266 WiFi模块:
使用ESP8266WiFi库初始化ESP8266 WiFi模块:
```
#include <ESP8266WiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
}
void loop() {
// 发送数据
WiFiClient client;
if (client.connect("www.example.com", 80)) {
client.println("GET /index.html HTTP/1.1");
client.println("Host: www.example.com");
client.println("Connection: close");
client.println();
while (client.connected()) {
if (client.available()) {
Serial.write(client.read());
}
}
client.stop();
}
delay(1000);
}
```
这些代码只是示例,您需要根据您使用的无线电和库进行必要的更改。
Arduino is ready. [INFO] [1683984140.630143]: Connected to Arduino on port /dev/ttyUSB0 at 115200 baud [INFO] [1683984140.651436]: arduino_led {'direction': 'output', 'type': 'Digital', 'rate': 5, 'pin': 13} published on topic /arduino/sensor/arduino_led Updating PID parameters Traceback (most recent call last): File "/home/nano/my_ws/src/ros_arduino_bridge/ros_arduino_python/nodes/arduino_node.py", line 226, in <module> myArduino = ArduinoROS() File "/home/nano/my_ws/src/ros_arduino_bridge/ros_arduino_python/nodes/arduino_node.py", line 142, in __init__ self.myBaseController = BaseController(self.controller, self.base_frame, self.name + "_base_controller") File "/home/nano/my_ws/src/ros_arduino_bridge/ros_arduino_python/src/ros_arduino_python/base_controller.py", line 59, in __init__ self.ticks_per_meter = self.encoder_resolution * self.gear_reduction / (self.wheel_diameter * pi) TypeError: can't multiply sequence by non-int of type 'float'
这个错误是因为在 base_controller.py 文件的第 59 行中,有一个除法运算的结果是 float 类型,而在同一行中还有一个整数乘法运算。这两个运算的结果类型不同,导致了错误。可能的解决方法是将整数转换为浮点数,例如:
```
self.ticks_per_meter = float(self.encoder_resolution * self.gear_reduction) / (self.wheel_diameter * pi)
```
这样就可以避免类型不匹配的错误了。
阅读全文
相关推荐
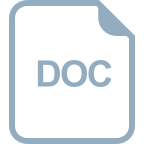
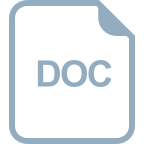
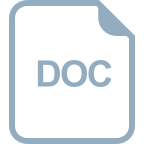
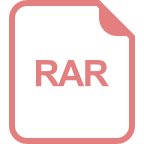
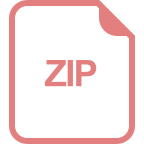
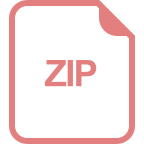
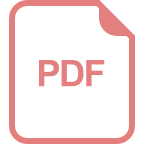
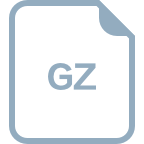








