c++编一个简单链表
时间: 2024-10-14 08:02:53 浏览: 20
在C++中,我们可以创建一个简单的单向链表结构,它由节点构成,每个节点包含数据和指向下一个节点的指针。下面是一个基本的链表类的示例:
```cpp
#include <iostream>
// 链表节点定义
struct Node {
int data; // 节点存储的数据
Node* next; // 指向下一个节点的指针
Node(int value) { // 构造函数
data = value;
next = nullptr; // 初始时指针为空
}
};
// 链表类
class LinkedList {
private:
Node* head;
public:
LinkedList() { // 构造函数,初始化头指针为nullptr
head = nullptr;
}
// 在链表末尾添加节点
void append(int value) {
if (head == nullptr) {
head = new Node(value);
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node(value);
}
}
// 打印链表中的所有元素
void printList() {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
// 删除指定值的节点(如果存在)
void removeNode(int value) {
if (head == nullptr) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
Node* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
}
}
};
int main() {
LinkedList list;
list.append(10);
list.append(20);
list.append(30);
std::cout << "Original list: ";
list.printList();
list.removeNode(20);
std::cout << "After removing 20: ";
list.printList();
return 0;
}
```
这个例子展示了如何创建一个链表类,包括添加元素、打印链表和删除指定值的节点的基本操作。
阅读全文
相关推荐
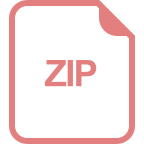
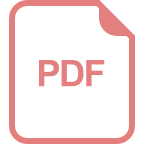
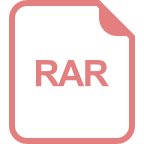
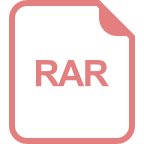
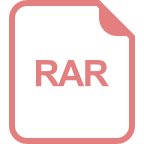

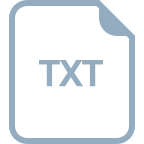
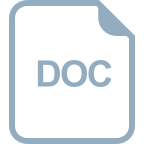
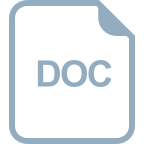
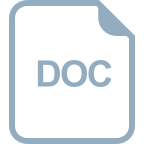
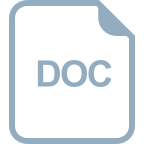
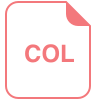






