编写Python代码,分别从给定的中文文本文件和英文文本中统计出最常出现的三个单词(中文单词长度大于等于2,英文单词长度大于等于5)。
时间: 2024-09-12 20:03:03 浏览: 42
要实现这一功能,我们需要完成以下步骤:
1. 读取文本文件内容。
2. 对文本内容进行预处理,包括分词、去除标点符号等。
3. 根据语言类型,统计每个单词出现的次数。
4. 找出出现次数最多的三个单词。
对于中文文本,可以使用jieba库进行分词,对于英文文本,可以使用空格和标点符号作为单词分隔符。以下是使用Python实现的示例代码:
```python
import jieba
import re
from collections import Counter
# 中文文本处理函数
def process_chinese(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
# 使用jieba进行分词
words = jieba.cut(content)
# 去除非中文字符
chinese_words = [word for word in words if re.match(r'[\u4e00-\u9fa5]', word) and len(word) >= 2]
# 统计词频
word_counts = Counter(chinese_words)
# 获取最常见的三个单词
most_common = word_counts.most_common(3)
return most_common
# 英文文本处理函数
def process_english(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
# 使用正则表达式分割单词
words = re.findall(r'\b\w+\b', content.lower())
# 筛选出长度大于等于5的单词
long_words = [word for word in words if len(word) >= 5]
# 统计词频
word_counts = Counter(long_words)
# 获取最常见的三个单词
most_common = word_counts.most_common(3)
return most_common
# 示例文件路径
chinese_file_path = 'path_to_chinese_text.txt'
english_file_path = 'path_to_english_text.txt'
# 处理中文文本
chinese_common_words = process_chinese(chinese_file_path)
print("中文文本中最常见的三个单词:", chinese_common_words)
# 处理英文文本
english_common_words = process_english(english_file_path)
print("英文文本中最常见的三个单词:", english_common_words)
```
在这段代码中,我们定义了两个函数`process_chinese`和`process_english`,分别用于处理中文和英文文本文件。我们使用`jieba`库来分词处理中文文本,而英文文本则通过正则表达式进行单词分割和筛选。最后,我们使用`collections.Counter`来统计词频并找出最常见的三个单词。
注意:在运行这段代码前,请确保已经安装了`jieba`库。如果没有安装,可以使用`pip install jieba`命令进行安装。
阅读全文
相关推荐
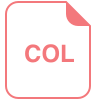
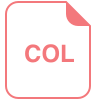
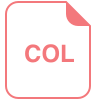
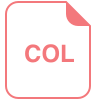
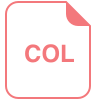
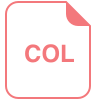
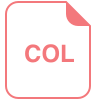
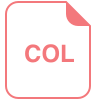

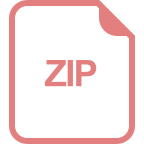
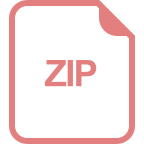
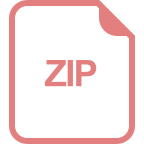
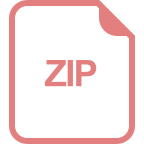
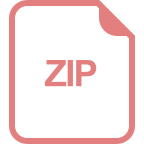
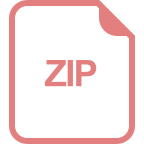
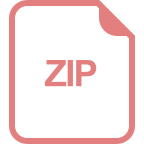
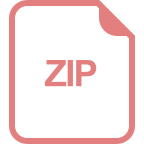