c语言哈希表解决冲突代码
时间: 2023-12-24 22:25:22 浏览: 86
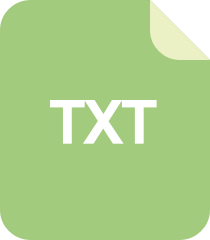
c语言hash table的实现

以下是C语言中使用链表法解决哈希表冲突的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define SIZE 10
// 哈希表节点结构体
struct Node {
int data;
struct Node* next;
};
// 哈希表结构体
struct HashTable {
struct Node** head;
int count;
};
// 创建哈希表
struct HashTable* createHashTable() {
struct HashTable* hashTable = (struct HashTable*)malloc(sizeof(struct HashTable));
hashTable->head = (struct Node**)malloc(sizeof(struct Node*) * SIZE);
hashTable->count = 0;
for (int i = 0; i < SIZE; i++) {
hashTable->head[i] = NULL;
}
return hashTable;
}
// 哈希函数
int hashFunction(int data) {
return data % SIZE;
}
// 插入数据
void insert(struct HashTable* hashTable, int data) {
int index = hashFunction(data);
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
if (hashTable->head[index] == NULL) {
hashTable->head[index] = node;
} else {
struct Node* p = hashTable->head[index];
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
hashTable->count++;
}
// 查找数据
int search(struct HashTable* hashTable, int data) {
int index = hashFunction(data);
struct Node* p = hashTable->head[index];
while (p != NULL) {
if (p->data == data) {
return 1;
}
p = p->next;
}
return 0;
}
// 删除数据
void delete(struct HashTable* hashTable, int data) {
int index = hashFunction(data);
struct Node* p = hashTable->head[index];
struct Node* pre = NULL;
while (p != NULL) {
if (p->data == data) {
if (pre == NULL) {
hashTable->head[index] = p->next;
} else {
pre->next = p->next;
}
free(p);
hashTable->count--;
return;
}
pre = p;
p = p->next;
}
}
// 打印哈希表
void printHashTable(struct HashTable* hashTable) {
for (int i = 0; i < SIZE; i++) {
printf("index %d: ", i);
struct Node* p = hashTable->head[i];
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
}
int main() {
struct HashTable* hashTable = createHashTable();
insert(hashTable, 1);
insert(hashTable, 11);
insert(hashTable, 21);
insert(hashTable, 31);
insert(hashTable, 2);
insert(hashTable, 12);
insert(hashTable, 22);
insert(hashTable, 32);
printHashTable(hashTable);
delete(hashTable, 21);
delete(hashTable, 31);
printHashTable(hashTable);
printf("search 11: %d\n", search(hashTable, 11));
printf("search 21: %d\n", search(hashTable, 21));
return 0;
}
```
阅读全文
相关推荐
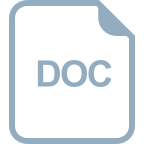
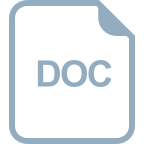
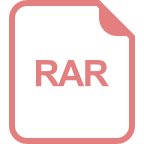
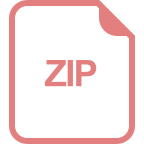
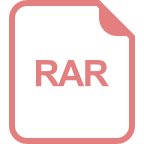
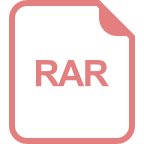
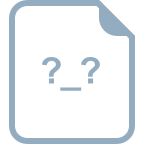
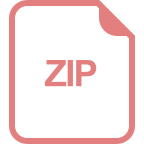
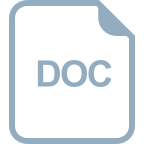
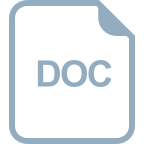
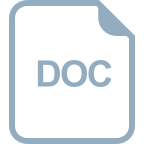
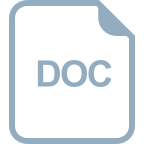





