如果是父组件调用子组件函数同时函数需要传入子组件参数,怎么办
时间: 2024-11-14 21:16:19 浏览: 15
当父组件需要调用子组件的函数,并传递子组件特有的数据作为参数时,可以采用以下几种常见的方法:
1. **props(属性)**:父组件可以通过props将数据传递给子组件。首先,在父组件中创建这个数据,然后作为prop传递给子组件。在子组件的函数签名中,接收这个prop作为参数。
```jsx
// 父组件
<ChildComponent data={parentData} />
// 子组件
function ChildComponent(props) {
function childFunction(childParam) {
// 使用props.data 和 childParam 进行操作
}
return <button onClick={() => childFunction(props.data)}>点击</button>;
}
```
2. **事件监听**:如果函数需要在特定触发时被调用,可以使用事件系统。父组件给子组件添加事件处理程序,该处理程序接受子组件的数据作为参数。
```jsx
// 父组件
<ChildComponent onFunction={(childParam) => ...} />
// 子组件
import { useRef } from 'react';
function ChildComponent({ onFunction }) {
const childRef = useRef();
function handleClick() {
onFunction(childRef.current.specialValue);
}
return <div ref={childRef} onClick={handleClick}>...</div>;
}
```
3. **回调函数**:通过回调函数的形式让子组件传递一个函数,该函数会在适当的时机接收到子组件的参数。
```jsx
// 父组件
function Parent() {
const callChildFunction = (childData) => {
// 调用子组件提供的函数
};
return <ChildComponent callback={callChildFunction} />;
}
// 子组件
function ChildComponent({ callback }) {
function childMethod(childParam) {
callback(childParam);
}
return <button onClick={() => childMethod(specialValue)}>点击</button>;
}
```
阅读全文
相关推荐
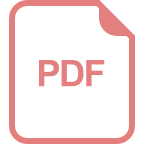
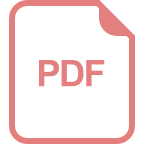
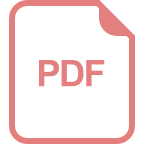
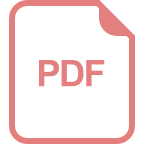
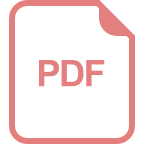
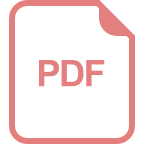
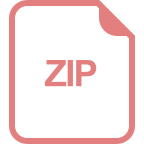
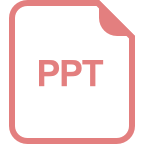
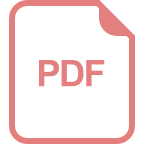
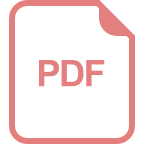
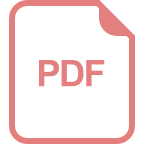
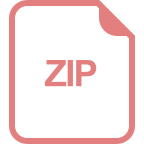
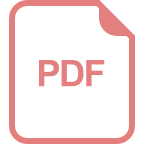
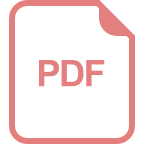
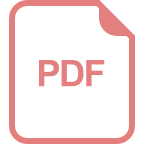
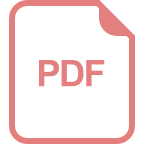
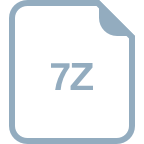
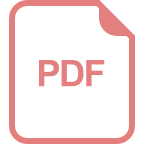

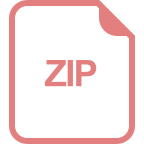