springboot集成操作ES
时间: 2023-09-17 22:06:41 浏览: 136
对于Spring Boot与Elasticsearch的集成,可以按照以下步骤进行操作:
1. 添加依赖:在`pom.xml`文件中添加Spring Data Elasticsearch的依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
```
2. 配置连接信息:在`application.properties`文件中配置Elasticsearch的连接信息。
```properties
spring.data.elasticsearch.cluster-name=your-cluster-name
spring.data.elasticsearch.cluster-nodes=localhost:9300
```
3. 创建实体类:创建与Elasticsearch索引对应的实体类,使用`@Document`注解指定索引名称和类型。
```java
@Document(indexName = "your-index-name", type = "your-type")
public class YourEntity {
// 实体类属性和Elasticsearch索引字段的映射关系
}
```
4. 创建Repository:创建继承自`ElasticsearchRepository`的接口,用于操作Elasticsearch索引。
```java
public interface YourEntityRepository extends ElasticsearchRepository<YourEntity, Long> {
// 可以自定义查询方法
}
```
5. 进行CRUD操作:通过调用自动生成的Repository方法,进行数据的增删改查操作。
```java
// 注入Repository
@Autowired
private YourEntityRepository yourEntityRepository;
// 插入数据
YourEntity entity = new YourEntity();
entity.setId(1L);
entity.setName("example");
yourEntityRepository.save(entity);
// 查询数据
Optional<YourEntity> result = yourEntityRepository.findById(1L);
if (result.isPresent()) {
YourEntity entity = result.get();
// 处理查询结果
}
// 更新数据
entity.setName("new example");
yourEntityRepository.save(entity);
// 删除数据
yourEntityRepository.delete(entity);
```
通过以上步骤,你就可以在Spring Boot中成功集成并操作Elasticsearch了。可以根据自己的具体需求,进一步扩展和优化相关功能。
阅读全文
相关推荐
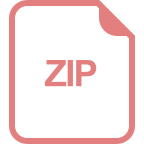














