用C语言编写创建2个线程的程序。父进程显示5次“Thisisthe parent,pidisxxxxxx”,每个线程显示5次“Thisisanewthread, threadIDisxxxxxx”。要求交叉显示父进程和子进程的显示内容。
时间: 2024-11-03 21:12:31 浏览: 27
在C语言中,我们可以使用POSIX线程库(pthread)来创建和管理线程。下面是一个简单的示例程序,展示了如何创建两个子线程并与父进程交替显示消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 2
#define THREAD_NAME "Thread"
#define MESSAGE_LEN 40
void *print_message(void *arg) {
char message[MESSAGE_LEN];
int id = (int)arg;
for (int i = 0; i < 5; i++) {
snprintf(message, sizeof(message), "%s, threadIDis%04d", THREAD_NAME, id);
printf("%s\n", message);
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[MAX_THREADS]; // 存储线程句柄
int thread_ids[] = {1, 2}; // 线程ID
char parent_msg[MESSAGE_LEN] = "This is the parent, pid is";
// 创建并启动子线程
for (int i = 0; i < MAX_THREADS; i++) {
sprintf(parent_msg + strlen(parent_msg), "%04d", getpid());
printf("%s\n", parent_msg);
if (pthread_create(&threads[i], NULL, print_message, (void *)thread_ids[i]) != 0) {
perror("Error creating thread");
return -1;
}
}
// 等待所有子线程完成
for (int i = 0; i < MAX_THREADS; i++) {
if (pthread_join(threads[i], NULL) != 0) {
perror("Error joining thread");
}
}
printf("\nAll threads finished.\n");
return 0;
}
```
这个程序首先定义了父进程和子线程的消息模板,然后在`main`函数里,我们创建了两个线程,并分别传入不同的线程ID。每次循环中,先打印父进程的消息,然后创建子线程。子线程会接收到线程ID并在内部打印出包含线程ID的消息。主线程等待所有的子线程完成后关闭。
阅读全文
相关推荐

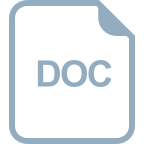
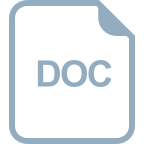
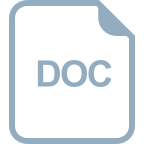
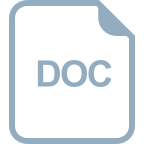


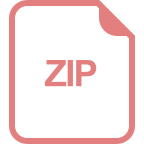
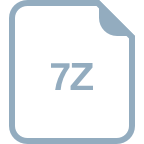
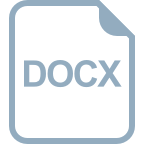
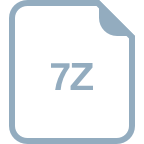
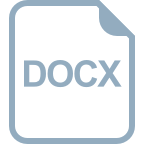