用C语言编写创建2个线程的程序。父进程显示5次“Thisisthe parent,pidisxxxxxx”,每个线程显示5次“Thisisanewthread, threadIDisxxxxxx”。要
时间: 2024-11-19 14:36:04 浏览: 26
在C语言中,我们可以使用POSIX线程库(pthread.h)来创建和管理线程。下面是一个简单的示例,展示了如何创建两个线程并让它们交替输出信息:
```c
#include <stdio.h>
#include <pthread.h>
#define MAX_THREADS 2
#define THREAD_NAME "NewThread"
#define PARENT_MSG "This is the parent, pid is %d\n"
void *thread_func(void *arg) {
int thread_id = (int)arg;
for (int i = 0; i < 5; i++) {
printf("This is a new thread, thread ID is %d\n", thread_id);
}
pthread_exit(NULL); // 线程结束时主动退出
}
int main() {
pthread_t threads[MAX_THREADS];
int thread_ids[] = {0, 1}; // 子线程编号
// 创建并启动线程
for (int i = 0; i < MAX_THREADS; i++) {
pthread_create(&threads[i], NULL, thread_func, (void*)thread_ids[i]);
}
// 显示父进程消息
for (int i = 0; i < 5; i++) {
printf(PARENT_MSG, getpid());
sleep(1); // 暂停一秒,让线程有时间运行
}
// 等待所有子线程完成
for (int i = 0; i < MAX_THREADS; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
在这个程序中,`main`函数首先创建了两个线程,然后循环打印五次父进程的消息,并在每次之间暂停一秒钟,以便让线程有机会运行。每个线程接收一个不同的`thread_id`作为参数,在`thread_func`内部输出相应的信息。
注意:这个例子需要支持POSIX线程API的环境才能运行,并且使用了`sleep`函数来控制线程的执行顺序,实际应用中可能需要更复杂的同步机制来达到期望的效果。
阅读全文
相关推荐

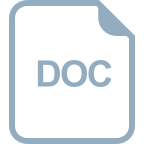
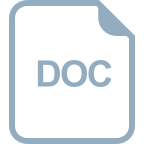
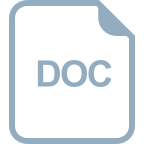
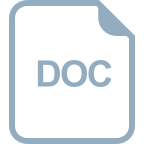


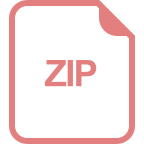
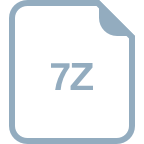
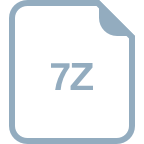
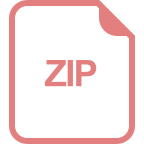