用C语言编写创建2个线程的程序。父进程显示5次“Thisisthe parent,pidisxxxxxx”,每个线程显示5次“Thisisanewthread, threadIDisxxxxxx”。要求交叉显示父进程和子进程的显示内容。
时间: 2024-11-07 07:23:46 浏览: 14
在C语言中,我们可以使用POSIX线程库(pthreads)来创建线程。下面是一个简单的示例,展示了如何创建一个父进程和两个子线程,它们交替打印出消息:
```c
#include <stdio.h>
#include <pthread.h>
#define MAX_MSGS 5
void *thread_function(void *arg) {
int id = (int)arg;
char msg[40] = "This is a new thread, threadID is ";
for (int i = 0; i < MAX_MSGS; ++i) {
snprintf(msg + strlen(msg), sizeof(msg) - strlen(msg), "%d", id);
printf("%s%dx\n", msg, i+1); // 每次循环后加1到id
pthread_yield(); // 为了控制打印顺序,让其他线程有机会运行
}
return NULL;
}
int main() {
pthread_t tid1, tid2; // 创建线程的句柄
int pid = getpid(); // 获取当前进程ID
// 创建并启动第一个线程
if (pthread_create(&tid1, NULL, thread_function, (void*)1)) {
perror("Failed to create thread 1");
exit(1);
}
// 创建并启动第二个线程
if (pthread_create(&tid2, NULL, thread_function, (void*)2)) {
perror("Failed to create thread 2");
exit(1);
}
// 父进程开始打印消息
for (int i = 0; i < MAX_MSGS; ++i) {
printf("This is the parent, process ID is %d, iteration %dx\n", pid, i+1);
pthread_yield();
}
// 等待子线程结束
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
printf("Parent process finished.\n");
return 0;
}
```
在这个例子中,`thread_function`被传递一个整数作为参数,表示线程的标识符。父进程和两个子线程轮流打印消息,通过`pthread_yield()`函数让线程之间切换。
阅读全文
相关推荐
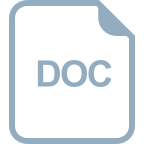
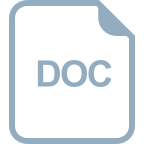
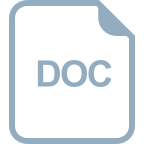

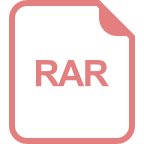
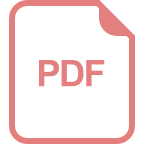
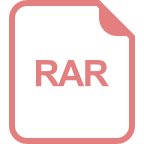
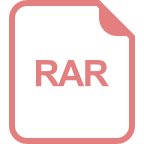
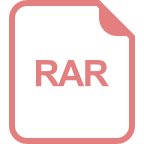
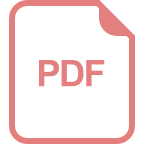
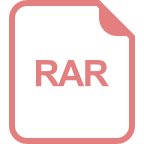
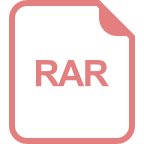