使用管道/过滤器风格设计(java语言),实现上下文关键字(KWIC)检索系统: 系统接受多行输入 每行有若干词 每个词由若干字符组成 每行都可以循环移位:重复地把第一个词删除,然后接到行末 KWIC把所有行的各种移位情况按照字母表顺序输出
时间: 2024-10-13 16:05:19 浏览: 167
在Java中,为了实现基于管道/过滤器风格的设计,我们通常可以采用流(Stream) API以及迭代器(Iterator)来构建一个简单的KWIC (Key-Word In Context) 检索系统。以下是步骤和伪代码概述:
1. 定义数据结构:首先,我们可以创建一个`KeywordWithContext`类,它包含关键词、其在原始文本中的位置以及上下文信息。
```java
public class KeywordWithContext {
private String keyword;
private int position;
private String context;
// getters and setters...
}
```
2. 输入处理:使用`Scanner`读取多行输入,并将每一行切割成单词,存储在一个列表中。
```java
List<String> words = new ArrayList<>();
Scanner scanner = new Scanner(System.in);
while (scanner.hasNextLine()) {
List<String> lineWords = Arrays.asList(scanner.nextLine().split("\\s+"));
words.addAll(lineWords);
}
```
3. 移位操作:使用一个自定义的迭代器,每次迭代都将第一个词移到末尾并更新`KeywordWithContext`实例的位置。
```java
class ShiftingIterator implements Iterator<KeywordWithContext> {
private Iterator<String> wordIterator;
private int currentIndex;
public ShiftingIterator(List<String> words) {
this.wordIterator = words.iterator();
currentIndex = -1;
}
@Override
public boolean hasNext() {
// 实现移位逻辑...
}
@Override
public KeywordWithContext next() {
// 更新位置和上下文...
}
}
```
4. KWIC生成:遍历所有移位后的结果,并按字母顺序排序输出。
```java
List<KeywordWithContext> kwicList = new ArrayList<>();
ShiftingIterator shiftingIter = new ShiftingIterator(words);
// 添加移位到kwicList
while (shiftingIter.hasNext()) {
kwicList.add(shiftingIter.next());
}
Collections.sort(kwicList, Comparator.comparing(KeywordWithContext::getKeyword));
for (KeywordWithContext kwic : kwicList) {
System.out.println(kwic.getKeyword() + " (" + kwic.getPosition() + "): " + kwic.getContext());
}
```
阅读全文
相关推荐
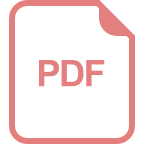
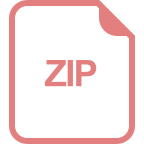
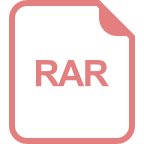











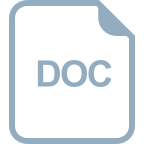
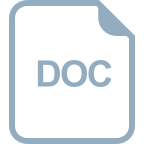
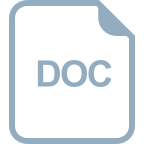
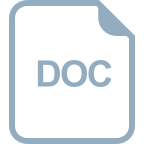
