java消消乐小游戏代码
时间: 2024-06-02 15:04:18 浏览: 212
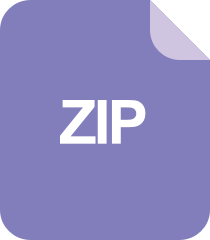
使用Java开发的消消乐小游戏.zip
Java消消乐小游戏的实现大致需要以下步骤:
1. 绘制游戏界面。使用Java Swing或JavaFX等GUI框架,绘制游戏的背景、方块、分数等界面元素。
2. 随机生成方块。使用Java的随机数函数,随机生成不同颜色的方块。
3. 处理用户操作。监听用户的鼠标点击事件,判断用户点击的方块是否符合消除条件,如果符合则进行消除操作。
4. 消除方块。使用递归或广度优先搜索等算法,寻找符合消除条件的方块并进行消除操作。
5. 计算得分。根据消除的方块数量和连击次数计算得分,并在界面上显示出来。
6. 判断游戏是否结束。如果屏幕上没有可消除的方块,则判断游戏结束,显示得分并提示用户是否重新开始游戏。
以下是一个简单的Java消消乐小游戏代码示例,仅供参考:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
public class XOXOGame extends JFrame implements MouseListener {
private JPanel panel;
private JLabel scoreLabel;
private int score;
private int[][] blocks;
private int blockCount;
public XOXOGame() {
panel = new JPanel(new GridLayout(8, 8));
panel.addMouseListener(this);
scoreLabel = new JLabel("Score: 0");
blocks = new int;
blockCount = 0;
initGame();
add(panel, BorderLayout.CENTER);
add(scoreLabel, BorderLayout.SOUTH);
setSize(500, 500);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void initGame() {
Random random = new Random();
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
blocks[i][j] = random.nextInt(5) + 1;
JLabel label = new JLabel(getBlockIcon(blocks[i][j]));
label.setHorizontalAlignment(JLabel.CENTER);
panel.add(label);
}
}
blockCount = 64;
}
private void updateScore(int count) {
score += count * count * 10;
scoreLabel.setText("Score: " + score);
}
private ImageIcon getBlockIcon(int type) {
String filename = "block_" + type + ".png";
return new ImageIcon(getClass().getResource(filename));
}
private boolean isValidBlock(int x, int y) {
return x >= 0 && x < 8 && y >= 0 && y < 8 && blocks[x][y] > 0;
}
private int findConnectedBlocks(int x, int y, int type) {
if (!isValidBlock(x, y) || blocks[x][y] != type) {
return 0;
}
blocks[x][y] = -1;
return 1 + findConnectedBlocks(x - 1, y, type)
+ findConnectedBlocks(x + 1, y, type)
+ findConnectedBlocks(x, y - 1, type)
+ findConnectedBlocks(x, y + 1, type);
}
private void removeConnectedBlocks(int x, int y, int type) {
if (!isValidBlock(x, y) || blocks[x][y] != -1) {
return;
}
blocks[x][y] = 0;
panel.remove(8 * x + y);
blockCount--;
updateScore(1);
removeConnectedBlocks(x - 1, y, type);
removeConnectedBlocks(x + 1, y, type);
removeConnectedBlocks(x, y - 1, type);
removeConnectedBlocks(x, y + 1, type);
}
private void handleMouseClicked(MouseEvent e) {
if (blockCount <= 0) {
JOptionPane.showMessageDialog(this, "Game Over! Score: " + score);
System.exit(0);
}
JLabel label = (JLabel) e.getComponent();
int index = panel.getComponentZOrder(label);
int x = index / 8;
int y = index % 8;
if (blocks[x][y] <= 0) {
return;
}
int type = blocks[x][y];
int count = findConnectedBlocks(x, y, type);
if (count < 2) {
return;
}
removeConnectedBlocks(x, y, type);
while (true) {
boolean flag = false;
for (int i = 7; i >= 0; i--) {
for (int j = 0; j < 8; j++) {
if (blocks[i][j] == 0 && isValidBlock(i - 1, j)) {
flag = true;
blocks[i][j] = blocks[i - 1][j];
blocks[i - 1][j] = 0;
panel.remove(8 * i + j);
JLabel newLabel = new JLabel(getBlockIcon(blocks[i][j]));
newLabel.setHorizontalAlignment(JLabel.CENTER);
panel.add(newLabel, 8 * i + j);
}
}
}
if (!flag) {
break;
}
try {
Thread.sleep(100);
} catch (InterruptedException ex) {}
panel.updateUI();
}
}
public void mouseClicked(MouseEvent e) {
handleMouseClicked(e);
}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public static void main(String[] args) {
new XOXOGame();
}
}
```
阅读全文
相关推荐
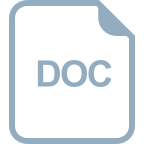

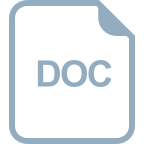
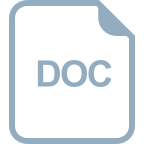
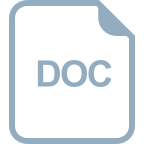







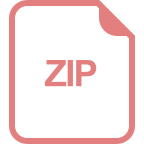


