输入年月,输出该年月的天数。 【输入形式】 输入的年月是合理的(0≤年份≤10000,1≤月份≤12), 如不合理输出"Error!", 注意格式:Error!后不包含换行符、回车符。 【输出形式】 输出为相应的数字表示,结尾处不包含换行符、回车符。
时间: 2024-11-18 13:27:28 浏览: 17
要实现这个功能,你需要根据每个月的天数规则来计算给定年月的天数。这里是一段简单的 C++ 代码示例,用于处理这种需求:
```cpp
#include <iostream>
// 定义一个结构体或类来存储月份和对应的天数
struct MonthDays {
int month;
int days;
};
// 填充每个月份的天数
MonthDays months[] = {
{1, 31}, // January
{2, 28}, // February (non-leap year)
{3, 31},
{4, 30},
{5, 31},
{6, 30},
{7, 31},
{8, 31},
{9, 30},
{10, 31},
{11, 30},
{12, 31} // December
};
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int get_days_in_month(int year, int month) {
if (year < 0 || year > 10000 || month < 1 || month > 12) {
return "Error!";
}
// Check for leap year and adjust February's days accordingly
if (month == 2 && is_leap_year(year)) {
return 29;
} else {
return months[month - 1].days;
}
}
int main() {
int year, month;
std::cout << "Enter year and month (separated by space): ";
std::cin >> year >> month;
// Call the function and output the result
int days = get_days_in_month(year, month);
if (days == "Error!") {
std::cerr << days; // Output error directly to standard error
} else {
std::cout << days;
}
return 0;
}
```
**相关问题--:**
1. 输入的年月如何检查其合理性?
2. 如何确定闰年的条件?
3. `get_days_in_month` 函数为什么要检查月份范围?
4. 为何要在 `main` 函数里分别对待错误结果和正常输出?
阅读全文
相关推荐
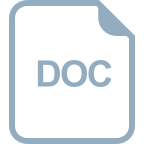
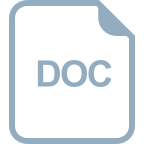
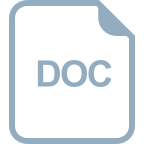

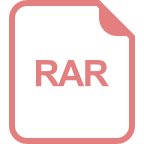













