编写一组程序,基于单链表,实现一元多项式的加法运算。多项式加法举例: p1=3x^3+5x^2+4x p2=×^5+3x^2 p1+p2=x^5+3x^3+8x^2+4x 输入:从大到小依次输入所要输入的两个一元多项式的系数和指数
时间: 2024-12-13 17:20:56 浏览: 17
为了编写一组程序,用于一元多项式的加法,我们可以创建一个Node类表示多项式的项,然后构建两个链表分别存储两个多项式的系数和指数。以下是使用Python语言的一个简单示例:
```python
class ListNode:
def __init__(self, coefficient, exponent):
self.coefficient = coefficient
self.exponent = exponent
self.next = None
def add_coefficients(coeff1, coeff2):
result = 0
if coeff1 > 0 and coeff2 > 0:
result = coeff1 + coeff2
elif coeff1 < 0 and coeff2 < 0:
result = coeff1 - coeff2
else:
result = max(coeff1, coeff2) # 如果一个为零,另一个非零,则取绝对值大的那个作为结果
return abs(result)
def polynomial_addition(list1, list2):
head = ListNode(0, 0)
current = head
carry = 0
while list1 is not None or list2 is not None:
if list1 is not None:
term1_coeff = list1.coefficient
list1 = list1.next
else:
term1_coeff = 0
if list2 is not None:
term2_coeff = list2.coefficient
list2 = list2.next
else:
term2_coeff = 0
product = add_coefficients(term1_coeff, term2_coeff) + carry
current.next = ListNode(product % 10, 1 if product >= 10 else 0) # 如果当前位数大于等于10,进位一位
carry = product // 10
current = current.next
# 将最后可能存在的进位处理在链表末尾
if carry > 0:
current.next = ListNode(carry, 1)
return head.next
# 示例输入
list1 = ListNode(3, 3) # 3x^3
list1.next = ListNode(5, 2) # 5x^2
list1.next.next = ListNode(4, 1) # 4x
list2 = ListNode(0, 5) # x^5 (0系数可以忽略)
list2.next = ListNode(3, 2) # 3x^2
# 加法操作
result = polynomial_addition(list1, list2)
```
在这个例子中,我们首先创建了`ListNode`类来存储每个多项式的系数和指数。`polynomial_addition`函数逐项相加,并处理进位。最后返回的是加法后的链表头部。
阅读全文
相关推荐








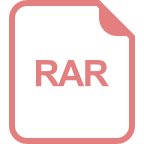


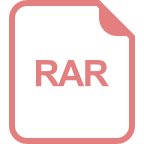



