使用c语言完成以下操作:输入三行字符串,第一行是主串,第二行是主串一个子串,最后输出更换完毕的字符串
时间: 2024-10-21 14:11:33 浏览: 14
在C语言中,你可以通过动态内存分配、字符串函数以及字符数组来完成这个操作。下面是一个简单的示例程序,它会从用户那里获取主串和子串,并替换所有在主串中找到的子串。
```c
#include <stdio.h>
#include <string.h>
#define MAX_STR_LEN 100 // 设置字符串最大长度
int main() {
char main_str[MAX_STR_LEN], sub_str[MAX_STR_LEN], result[MAX_STR_LEN * 2]; // 定义三个字符串变量
printf("请输入主串: ");
fgets(main_str, sizeof(main_str), stdin); // 输入主串,fgets处理换行符
main_str[strcspn(main_str, "\n")] = '\0'; // 删除末尾的换行符
printf("请输入子串: ");
fgets(sub_str, sizeof(sub_str), stdin);
sub_str[strcspn(sub_str, "\n")] = '\0';
printf("请输入要替换成的新字符串: ");
fgets(result, sizeof(result), stdin);
result[strcspn(result, "\n")] = '\0';
// 查找并替换子串
size_t pos = 0;
while ((pos = strstr(main_str + pos, sub_str)) != NULL) { // 使用strstr查找子串的位置
if (pos + strlen(sub_str) > strlen(main_str)) break; // 防止越界
strncpy(result + strlen(result), main_str + pos, strlen(sub_str)); // 复制子串到结果
result[strlen(result)] = '\0'; // 添加终止符
memmove(main_str + pos, main_str + pos + strlen(sub_str), strlen(main_str) - pos); // 移动主串内容
pos += strlen(sub_str); // 更新搜索位置
}
strncpy(result + strlen(result), main_str, strlen(main_str)); // 将剩余部分复制到结果
printf("替换后的字符串: %s\n", result);
return 0;
}
```
阅读全文
相关推荐
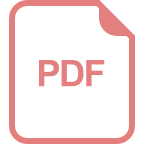
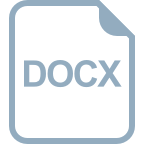
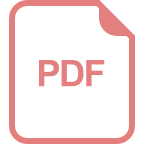




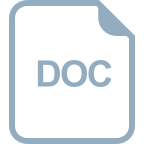
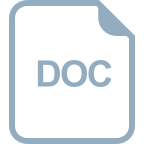
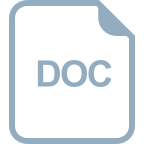
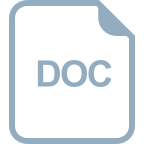
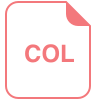
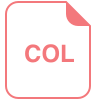


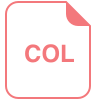


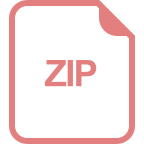
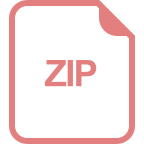