提供一个c语言实现的adpcm编解码算法,并提供测试demo
时间: 2024-10-15 20:12:13 浏览: 34
ADPCM (Adaptive Differential Pulse Code Modulation) 是一种音频编码技术,通过量化误差预测和自适应量化来压缩信号。在C语言中实现ADPCM编解码需要一些基本的数学运算和位操作。这里给出一个简单的线性预测和逐次逼近ADPCM编码解码的示例。请注意,这个代码只是一个简化的版本,实际应用可能会更复杂。
首先,我们有一个简单的ADPCM系数表(这里使用8位):
```c
const int adpcm_table[] = {7, 8, 9, 10, 11, 12, 13, 14, 16};
```
下面是ADPCM编码函数:
```c
void adpcm_encode(int *input, int *output, int frame_size, int table[], int step_size)
{
for (int i = 0; i < frame_size; i++)
{
if (*input > 0)
*output++ = *input - table[*input >> 4];
else
*output++ = table[(*input + step_size) >> 4];
*input <<= 1;
if (*input & 0x8000)
*input -= step_size;
}
}
```
然后是解码函数:
```c
void adpcm_decode(int *encoded, int *decoded, int frame_size, int table[], int step_size)
{
int sign = encoded[0] >> 7; // Get the sign bit
int sample = encoded[0] & 0x7f;
for (int i = 1; i < frame_size; i++)
{
int delta = encoded[i];
if (delta >= 0x80)
{
delta = - delta);
}
decoded[i] = (sample + delta) << 1;
if ((sample + delta) >= 0x8000)
{
decoded[i] += step_size;
}
sample = decoded[i];
}
decoded[frame_size - 1] &= ~sign; // Apply sign correction
}
```
为了演示,你可以创建一个主程序,先用编码函数处理输入数据,然后用解码函数恢复原始数据:
```c
int main()
{
// 示例数据
const int input[] = ...;
int encoded[frame_size], decoded[frame_size];
// 编码
adpcm_encode(input, encoded, frame_size, adpcm_table, 128);
// 解码
adpcm_decode(encoded, decoded, frame_size, adpcm_table, 128);
// 检查解码结果是否接近原数据
for (int i = 0; i < frame_size; i++)
{
printf("Original: %d, Decoded: %d\n", input[i], decoded[i]);
}
return 0;
}
```
阅读全文
相关推荐
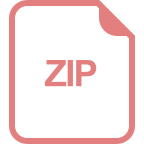
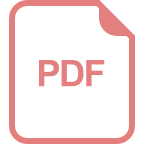
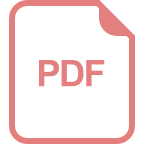
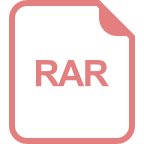
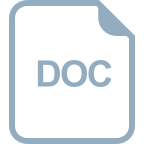
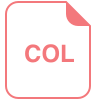


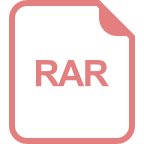
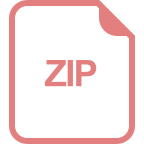
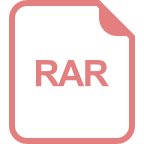
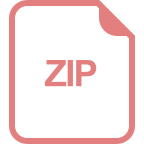
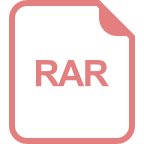
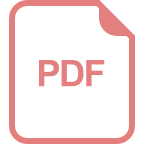
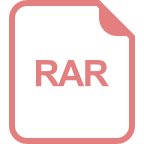
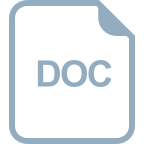


