如何在Python中实现模拟退火算法以优化组合问题?请结合代码示例进行详细解释。
时间: 2024-12-05 15:27:55 浏览: 18
模拟退火算法是一种强大的启发式搜索算法,特别适用于解决优化问题,如旅行商问题(TSP)、装箱问题等组合优化问题。在Python中实现模拟退火算法需要理解算法的基本原理和关键步骤,结合具体问题进行代码的编写和调整。
参考资源链接:[掌握模拟退火算法:Python实现详解](https://wenku.csdn.net/doc/7bv17fqr87?spm=1055.2569.3001.10343)
首先,需要定义问题的适应度函数,这通常是根据组合问题的目标函数来确定的。例如,在TSP问题中,适应度函数可以是路径长度的倒数,我们希望找到路径最短的解,即适应度值最大的解。
其次,初始化参数包括初始温度、冷却率、终止温度或迭代次数等。初始温度不宜过低,以保证算法有足够的机会跳出局部最优。冷却率决定了温度下降的速度,也会影响算法的性能和搜索效率。
在迭代搜索的过程中,我们需要在当前解的基础上产生一个新解,这可以通过随机扰动当前解来实现。接着,根据适应度函数计算新解的适应度,并决定是否接受新解。这通常通过一个接受概率函数来确定,接受概率会随着温度的降低而减小。
在Python中,可以使用随机模块来实现解的扰动和新解的接受判断。以下是一个简化的代码示例:
```python
import random
# 适应度函数示例(以最大化问题为例)
def fitness(solution):
# 根据具体问题定义适应度计算方法
return sum([cost_matrix[solution[i]][solution[(i+1)%len(solution)]] for i in range(len(solution))])
# 接受概率函数
def acceptance_probability(old_fitness, new_fitness, temperature):
if new_fitness > old_fitness:
return 1.0 # 如果新解更好,总是接受
else:
return math.exp((new_fitness - old_fitness) / temperature) # Metropolis准则
# 模拟退火算法主体
def simulated_annealing(initial_solution, initial_temperature, cooling_rate, final_temperature):
current_solution = initial_solution
current_fitness = fitness(current_solution)
temperature = initial_temperature
while temperature > final_temperature:
new_solution = perturb(current_solution) # 产生新解
new_fitness = fitness(new_solution)
if acceptance_probability(current_fitness, new_fitness, temperature) > random.random():
current_solution = new_solution # 接受新解
current_fitness = new_fitness
temperature *= cooling_rate # 降温处理
return current_solution, current_fitness
# 示例:使用模拟退火算法求解TSP问题
# cost_matrix为城市间距离矩阵
cost_matrix = [...]
initial_solution = [0]*len(cost_matrix) # 随机生成一个初始解
initial_temperature = 10000
cooling_rate = 0.995
final_temperature = 1
best_solution, best_fitness = simulated_annealing(initial_solution, initial_temperature, cooling_rate, final_temperature)
print(
参考资源链接:[掌握模拟退火算法:Python实现详解](https://wenku.csdn.net/doc/7bv17fqr87?spm=1055.2569.3001.10343)
阅读全文
相关推荐
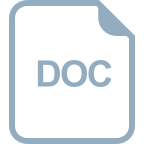
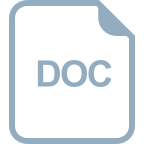
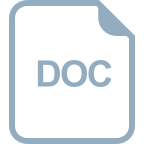
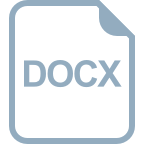
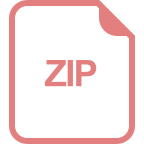
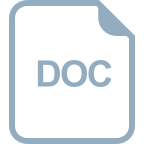
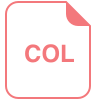
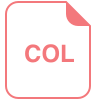
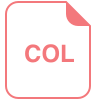
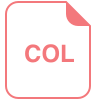
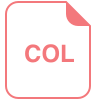
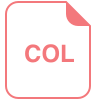
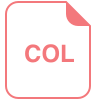
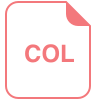
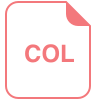
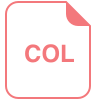

