C语言定义二叉树的链式存储结构; 实现二叉树的基本操作:建空树、销毁二叉树、生成二叉树(先序,中序或后序)、判二叉树是否为空、求二叉树的深度、求二叉树的根等基本算法; 实现二叉树的递归(先序、中序或后序)遍历算法。
时间: 2024-11-12 14:16:54 浏览: 66
在C语言中,我们可以使用结构体来定义二叉树的链式存储结构。下面是一个简单的二叉树节点定义示例:
```c
typedef struct TreeNode {
int val; // 节点值
struct TreeNode* left; // 左孩子指针
struct TreeNode* right; // 右孩子指针
} TreeNode;
```
以下是实现二叉树的一些基本操作:
1. **创建空树**:
```c
TreeNode* createEmptyTree() {
return NULL;
}
```
2. **销毁二叉树** (这里仅删除节点,不考虑内存管理):
```c
void destroyTree(TreeNode* root) {
if (root != NULL) {
destroyTree(root->left);
destroyTree(root->right);
free(root); // 根据实际情况处理内存释放
}
}
```
3. **生成特定顺序的二叉树** (如先序、中序或后序遍历构建):
- 先序遍历:根-左-右
```c
TreeNode* buildPreorder(int arr[], int n) {
// 递归实现...
}
```
- 中序遍历:左-根-右
```c
TreeNode* buildInorder(int arr[], int n) {
// 递归实现...
}
```
- 后序遍历:左-右-根
```c
TreeNode* buildPostorder(int arr[], int n) {
// 递归实现...
}
```
4. **判断二叉树是否为空**:
```c
bool isTreeEmpty(TreeNode* root) {
return root == NULL;
}
```
5. **计算二叉树的深度** (使用递归):
```c
int treeDepth(TreeNode* root) {
if (root == NULL) return 0;
return 1 + max(treeDepth(root->left), treeDepth(root->right));
}
```
6. **获取二叉树的根** (直接返回非空树的根):
```c
int getRootValue(TreeNode* root) {
return root->val;
}
```
7. **递归遍历** (例如先序遍历):
```c
void inorderTraversal(TreeNode* root) {
if (root != NULL) {
inorderTraversal(root->left);
printf("%d ", root->val); // 输出节点值
inorderTraversal(root->right);
}
}
```
阅读全文
相关推荐







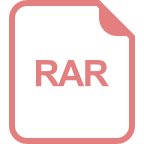
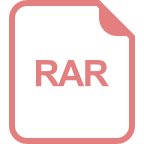

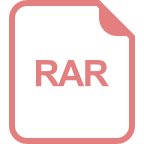

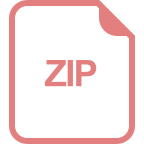