编写程序,输⼊⼀个数字,统计这个数的各个位上的数字总共出现了⼏次,最后按照从⼩到⼤进⾏输出。
时间: 2024-10-25 07:13:33 浏览: 13
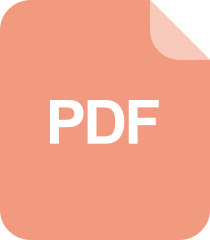
Python3实现个位数字和十位数字对调, 其乘积不变

当你编写这样的程序时,通常会采用循环遍历每一位数字,并将其存储在一个数据结构中,比如哈希表或字典,来记录每个数字出现的次数。以下是使用Python语言的一个简单示例:
```python
def count_digits(n):
digit_count = {} # 创建一个空的字典来存储各数字及其出现次数
while n > 0:
remainder = n % 10 # 取余数得到当前位的数字
if remainder in digit_count:
digit_count[remainder] += 1
else:
digit_count[remainder] = 1
n //= 10 # 向左移除一位
sorted_count = sorted(digit_count.items(), key=lambda x: x[0]) # 按照键值从小到大排序
return [digit for digit, count in sorted_count]
# 输入数字
number = int(input("请输入一个数字: "))
result = count_digits(number)
print("各个位上数字的出现次数,按从小到大顺序:")
for digit, count in result:
print(f"{digit}: {count}次")
阅读全文
相关推荐
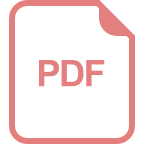
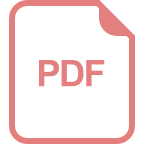









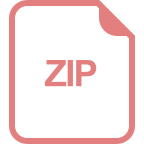
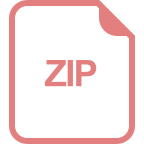
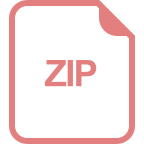
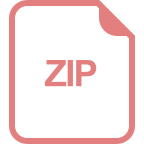
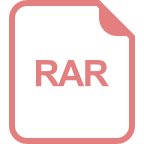