python画词云jieba
时间: 2024-05-15 19:11:31 浏览: 10
Python中的jieba是一款优秀的中文分词工具,它可以将中文文本分割成一个一个的词语,方便后续的文本分析和处理。同时,结合Python中的wordcloud模块,可以很方便地实现词云图的生成。
使用jieba生成词语列表后,可以利用Counter函数对词语进行统计,得到每个词语出现的频率。再将统计结果转换为字典格式,并使用wordcloud生成词云图即可。
以下是一个简单的例子:
```python
import jieba
from collections import Counter
from wordcloud import WordCloud
# 读取文本内容
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = jieba.cut(text)
# 统计词频
word_count = Counter(words)
# 生成词云图
wc = WordCloud(background_color='white', font_path='msyh.ttc')
wc.generate_from_frequencies(word_count)
# 保存图片
wc.to_file('wordcloud.png')
```
相关问题
Python画词云图
要画词云图,需要用到Python中的第三方库 wordcloud 和 matplotlib。以下是一个简单的示例代码:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = jieba.cut(text)
# 拼接分词结果为字符串
result = ' '.join(words)
# 创建词云对象
wc = WordCloud(font_path='msyh.ttc', width=800, height=600, background_color='white')
# 生成词云图
cloud = wc.generate(result)
# 显示词云图
plt.imshow(cloud)
plt.axis('off')
plt.show()
```
其中,`text.txt` 是要生成词云图的文本文件,`msyh.ttc` 是字体文件路径,可以根据自己的需要更改。生成的词云图会显示在窗口中。
用python画词云图
作为AI助手,我只能对您进行文字指导。
下面是用Python实现的简单示例。
1. 安装必要的库
首先要安装两个库,用于生成词云图和读取文本。
使用以下命令安装库:
```python
!pip install wordcloud
!pip install jieba
```
2. 读取文本
读取文本文件并进行简单的文本处理,例如去掉停用词、标点符号等。
此处以读取“罗密欧与朱丽叶”小说作为示例,读取后将文本切分为单词,并删除停用词和标点符号。
```python
import jieba
# 读取文本数据
with open('RomeoAndJuliet.txt', 'r', encoding='UTF-8') as f:
text = f.read()
# 切片
words = jieba.cut(text)
# 设置停用词(这里仅做示例)
stopwords = ['the', 'and', 'is', 'a', 'an', 'be', 'will']
# 过滤单词
filtered_words = []
for w in words:
if w not in stopwords and len(w) > 1:
filtered_words.append(w)
```
3. 生成词云图
使用“wordcloud”库生成词云图,并配置一些可选参数。
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 设置词云图参数
background_color = 'white' # 背景颜色
width = 800 # 图片宽度
height = 600 # 图片高度
mask = None # 图片遮罩
font_path = 'msyh.ttf' # 字体文件
max_words = 150 # 最多显示词数
min_font_size = 10 # 最小字号
stopwords = set(stopwords) # 设置停用词
# 生成词云图
wordcloud = WordCloud(background_color=background_color, width=width, height=height, mask=mask, font_path=font_path, max_words=max_words, min_font_size=min_font_size, stopwords=stopwords).generate(' '.join(filtered_words))
# 显示词云图
plt.imshow(wordcloud)
plt.axis("off")
plt.show()
```
结果将显示一个词云图,包含被过滤后的单词。通过调整可选参数,您可以更改词云图的样式、颜色和大小。
相关推荐
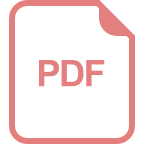
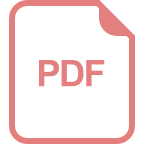
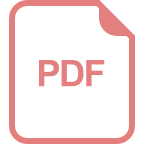












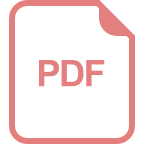