利用springboot-websocket 和Tomcat-websocket 实现websocket 客户端 并支持wss协议 ssl证书认证
时间: 2024-11-06 22:19:54 浏览: 29
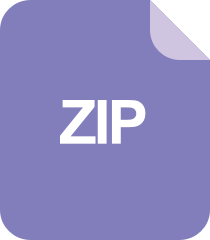
展示tio-websocket的用法,t-io官方提供的唯一tio-websocket示范教程,包括wss和监控等高级特性

在Spring Boot中利用WebSocket功能,通常会结合Spring Websocket库和WebSocket客户端库,例如`org.springframework.web.reactive.socket.client.WebSocketClient`,来实现实时通信。对于HTTPS(wss)的支持,需要配置SSL/TLS证书。
首先,你需要在Spring Boot项目中添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
然后,在`application.yml`或`application.properties`文件中配置WebSocket服务器地址和SSL相关的设置:
```yaml
server:
port: 8443 # 如果你的服务器在非标准端口运行
servlet:
context-path: /your-context-path
ssl:
key-store: classpath:keystore.jks
key-store-password: your-password
keyAlias: your-key-alias
spring:
application:
name: your-app-name
webflux:
websocket:
clients:
default:
uri: wss://your-backend-url/websocket
```
创建WebSocket客户端:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.http.HttpHeaders;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.stereotype.Component;
import org.springframework.util.Assert;
import org.springframework.web.reactive.function.client.WebClient;
@Component
public class WebSocketClient {
private final ApplicationContext context;
@Autowired
public WebSocketClient(ApplicationContext context) {
this.context = context;
}
public Mono<Void> sendMessage(String message) {
// 获取当前用户凭据
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 添加WS-Security header if needed (for SSL)
String user = auth.getName(); // 假设用户名已经从token解析出来
headers.set("Sec-WebSocket-Protocol", "your-custom-subprotocol");
headers.setBasicAuth(user, "your-password");
return WebClient.create(context.getBean("uri", URI.class))
.header(HttpHeaders.HOST, "your-backend-url")
.headers(headers)
.post()
.retrieve()
.bodyToMono(Void.class);
}
}
```
当客户端尝试连接时,Spring Security会对连接请求进行验证。如果证书有效并且连接到的是正确的URL,那么连接将成功并可以开始发送和接收消息。
阅读全文
相关推荐
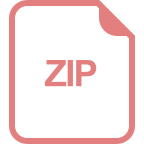
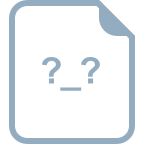
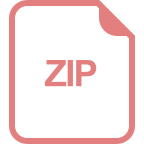
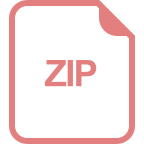
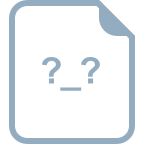
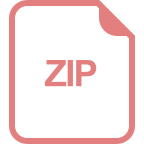
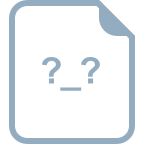
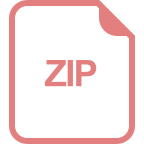
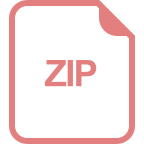
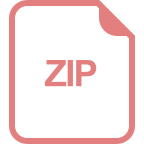
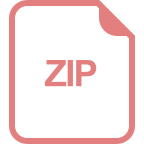
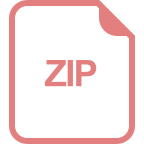
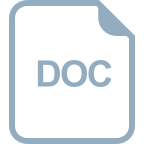




