stm32 字符串拼接
时间: 2023-10-31 16:57:00 浏览: 371
在STM32中进行字符串拼接可以使用标准C库函数或者自定义函数来实现。以下是两种方法的示例:
1. 使用标准C库函数:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello";
char str2[] = "World";
char result[30];
// 使用 strcat() 函数进行字符串拼接
strcat(result, str1);
strcat(result, " ");
strcat(result, str2);
printf("拼接结果: %s\n", result);
return 0;
}
```
2. 自定义函数:
```c
#include <stdio.h>
// 自定义函数实现字符串拼接
void stringConcat(char* str1, char* str2, char* result) {
int i = 0, j = 0;
// 复制第一个字符串到结果数组
while (str1[i] != '\0') {
result[i] = str1[i];
i++;
}
// 在第一个字符串后面添加空格
result[i] = ' ';
i++;
// 复制第二个字符串到结果数组
while (str2[j] != '\0') {
result[i] = str2[j];
i++;
j++;
}
// 结果数组最后加上字符串结束符
result[i] = '\0';
}
int main() {
char str1[20] = "Hello";
char str2[] = "World";
char result[30];
// 调用自定义函数进行字符串拼接
stringConcat(str1, str2, result);
printf("拼接结果: %s\n", result);
return 0;
}
```
无论是使用标准C库函数还是自定义函数,都可以实现字符串的拼接操作。选择哪种方法取决于你的喜好和特定的需求。
阅读全文
相关推荐
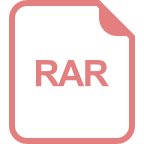


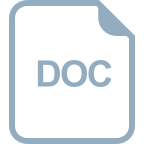








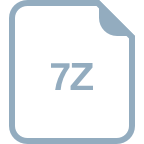
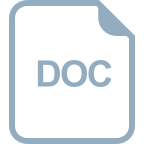


