在qt中使用unordered_map
时间: 2024-01-22 14:16:28 浏览: 162
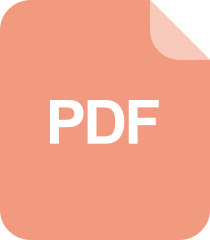
C++中的哈希容器unordered_map使用示例
在Qt中使用unordered_map可以通过包含头文件<unordered_map>来实现。unordered_map是一个无序的关联容器,它存储键值对,并且可以根据键快速查找值。下面是一个使用unordered_map的示例代码:
```cpp
#include <QApplication>
#include <unordered_map>
#include <iostream>
#include <QDebug>
int main(int argc, char *argv[]) {
QApplication a(argc, argv);
std::unordered_map<int, QString> map = {
{3, "fdd"},
{1, "fdd"},
{2, "fdd"}
};
for(auto k : map) {
qInfo() << k;
}
return 1;
}
```
在这个示例中,我们首先包含了<unordered_map>头文件,然后创建了一个unordered_map对象map,并使用{}语法进行初始化。然后,我们使用for循环遍历unordered_map中的所有键值对,并使用qInfo()函数打印出来。
注意:在使用unordered_map之前,确保已经包含了<unordered_map>头文件,并且使用了命名空间std。
阅读全文
相关推荐
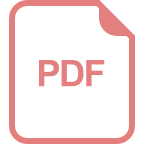







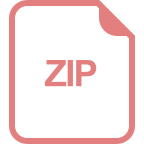
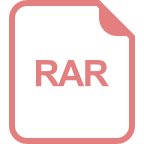
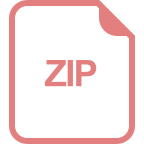
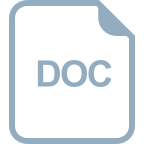
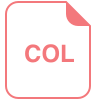
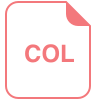


