ptorch实现MLP
时间: 2024-01-30 11:02:40 浏览: 58
以下是使用PyTorch实现多层感知机(MLP)的示例代码:
```python
import torch
import torch.nn as nn
# 定义多层感知机模型
class MLP(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_dim, hidden_dim)
self.fc2 = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# 定义输入数据
x = torch.randn(128, 20) # 输入的维度是(128,20)
# 定义MLP模型
model = MLP(input_dim=20, hidden_dim=30, output_dim=10)
# 计算输出
output = model(x)
# 打印输出的形状
print('output.shape:\n', output.shape)
```
在上面的代码中,我们首先定义了一个MLP类,该类继承自nn.Module类。在MLP类的构造函数中,我们定义了两个线性层(即全连接层),分别是fc1和fc2。在MLP类的前向函数中,我们首先使用ReLU激活函数对fc1的输出进行激活,然后将其输入到fc2中得到最终的输出。
接下来,我们定义了一个输入数据x,其维度为(128,20)。然后,我们创建了一个MLP模型,并将输入数据x输入到该模型中,得到输出output。最后,我们打印了输出的形状。
阅读全文
相关推荐



















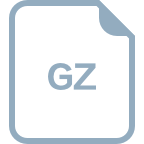