利用Python将Iris数据集输出得到条形图,不同特征用不同的颜色区分,对每个数据集的单个特征做 min-max 归一化,以类别名称为横坐标,以各个类别的特征的平均值为纵坐标,并标注每个类别中区分最大的两个特征的取值
时间: 2024-12-22 10:33:33 浏览: 8
在Python中,我们可以使用matplotlib和pandas库来创建这样的条形图。首先,我们需要导入必要的库,读取Iris数据集(通常使用sklearn库中的load_iris函数),然后进行数据预处理,包括归一化和计算平均值。以下是实现这个过程的一个步骤:
```python
# 导入所需库
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
from sklearn.preprocessing import MinMaxScaler
# 加载Iris数据集
iris = load_iris()
data = pd.DataFrame(data=np.c_[iris['data'], iris['target']], columns=iris.feature_names + ['class'])
# 对特定特征进行min-max归一化
scaler = MinMaxScaler()
data[['sepal length (cm)', 'sepal width (cm)', 'petal length (cm)', 'petal width (cm)']] = scaler.fit_transform(data[['sepal length (cm)', 'sepal width (cm)', 'petal length (cm)', 'petal width (cm)']])
# 计算每个类别的平均值
grouped_data = data.groupby('class').mean()
# 创建条形图,类别名作为x轴,平均值作为y轴
plt.figure(figsize=(10,6))
for feature in grouped_data.columns[:-1]: # 不绘制类别列
x = grouped_data.index.values
y = grouped_data[feature]
plt.bar(x, y, label=feature, color=plt.cm.get_cmap('tab10', len(iris.target_names))([i for i in range(len(iris.target_names))])) # 使用不同的颜色
# 添加最大最小值的标注
max_values = data.groupby(['class'])[['sepal length (cm)', 'sepal width (cm)', 'petal length (cm)', 'petal width (cm)']].max().reset_index()
for i, row in max_values.iterrows():
for feature, value in zip(['sepal length (cm)', 'sepal width (cm)', 'petal length (cm)', 'petal width (cm)'], row[-4:].tolist()):
plt.annotate(f'Max {value} for {row["class"]}: {feature}', xy=(i, value), textcoords='offset points')
# 设置标题、标签、图例等
plt.title("Average Feature Values of Iris Classes")
plt.xlabel('Class')
plt.ylabel('Normalized Value')
plt.xticks(range(4), iris.target_names)
plt.legend(title="Features", loc='upper right')
plt.show()
```
阅读全文
相关推荐
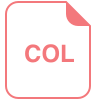
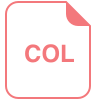
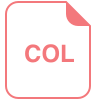
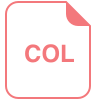
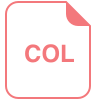
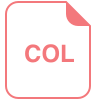
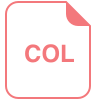
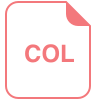
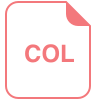
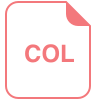
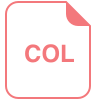
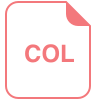
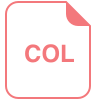
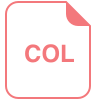
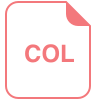
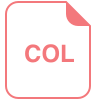
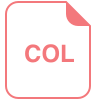
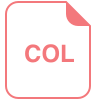
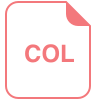