std::thread+设置线程优先级
时间: 2023-12-13 13:33:55 浏览: 469
可以使用std::thread::native_handle()函数获取线程的本地句柄,然后使用操作系统提供的函数设置线程的优先级。例如,在Windows操作系统中,可以使用SetThreadPriority()函数设置线程的优先级。下面是一个示例代码:
```c++
#include <iostream>
#include <thread>
#include <windows.h>
void thread_func()
{
std::cout << "Thread running" << std::endl;
}
int main()
{
std::thread t(thread_func);
HANDLE handle = t.native_handle();
SetThreadPriority(handle, THREAD_PRIORITY_HIGHEST);
t.join();
return 0;
}
```
在上面的代码中,我们创建了一个std::thread对象t,并将其关联到函数thread_func。然后,我们使用t.native_handle()函数获取线程的本地句柄,并使用SetThreadPriority()函数将线程的优先级设置为THREAD_PRIORITY_HIGHEST。最后,我们使用t.join()函数等待线程执行完毕。
相关问题
std::thread 创建线程,设置优先级
`std::thread` 是 C++ 标准库中的一个类,用于创建和管理线程。在 C++11 及以后版本中,你可以使用它来启动一个新的线程并指定线程执行的函数或成员函数。
创建线程的基本步骤如下:
```cpp
#include <thread>
// 定义要在线程中运行的函数
void my_function() {
// 线程体
}
int main() {
// 创建线程并传入函数指针作为参数
std::thread thread_instance(my_function);
// 如果需要,可以立即启动线程
thread_instance.start();
// 主线程继续执行其他任务
// 通常线程会等到join()调用完成后再退出
thread_instance.join(); // 等待线程结束
return 0;
}
```
关于线程优先级的设置,在 C++17 及以上版本里,你可以使用 `std::thread::priority` 属性。然而,这并不是标准的一部分,也不是所有系统都支持。C++11 和之前的版本通常通过操作系统提供的接口来控制线程优先级,例如 Windows 的 `_beginthreadex` 或 Posix 的 `pthread_setschedparam`,但是这需要操作系统的配合,并不是跨平台的标准做法。
在 C++11 中,如果你想要改变线程的优先级,可能需要依赖于特定的操作系统API,或者使用第三方库如 Boost.Thread。不过,通常情况下,程序员更倾向于让操作系统自动调度线程,而不是直接干预,因为操作系统有更复杂的调度算法来优化性能。
std::thread 优先级
std::thread是C++11及之后版本中的多线程编程方式之一,它可以通过std::thread::native_handle()函数获取线程的本地句柄,然后使用操作系统提供的API来修改线程的优先级。下面是一个例子,展示了如何使用std::thread来创建线程,并修改线程的优先级:
```c++
#include <iostream>
#include <thread>
#include <chrono>
#include <pthread.h>
void thread_func()
{
std::cout << "Thread running..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
int main()
{
std::thread t(thread_func);
// 获取线程的本地句柄
pthread_t native_handle = t.native_handle();
// 设置线程的调度策略和优先级
sched_param sch_params;
sch_params.sched_priority = 10;
int policy = SCHED_FIFO;
pthread_setschedparam(native_handle, policy, &sch_params);
t.join();
return 0;
}
```
在上面的例子中,我们创建了一个std::thread对象t,并将其绑定到函数thread_func上。然后,我们使用t.native_handle()函数获取线程的本地句柄,并使用pthread_setschedparam()函数来修改线程的调度策略和优先级。在这个例子中,我们将线程的优先级设置为10,调度策略设置为SCHED_FIFO。
阅读全文
相关推荐
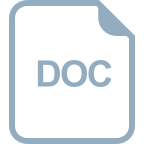
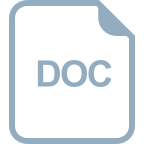
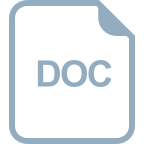
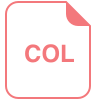
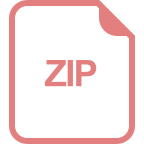
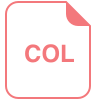
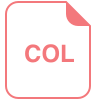

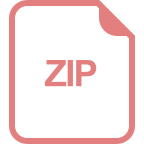
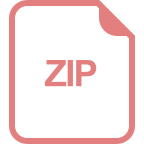
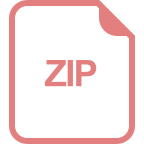
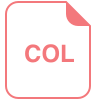
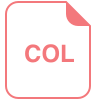
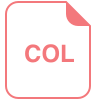
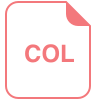
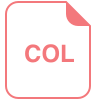
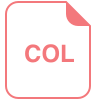