已知模式串t=‘abcaabbabcab’编程写出完整的KMP算法、并输出每个字符对应的next函数值和nextval函数值c语言编程上机实验代码算法主要部分要求有注释
时间: 2024-11-18 08:28:01 浏览: 51
KMP(Knuth-Pratt)算法是一种用于字符串匹配的有效算法,尤其对于处理长文本搜索的问题。下面是使用C语言实现KMP算法的一部分核心代码,包括计算next函数值(存储前缀函数)的过程:
```c
#include <stdio.h>
#include <string.h>
// 计算next数组,用于存储前缀函数
int computeNext(char* pattern) {
int len = strlen(pattern);
int next[len];
next[0] = -1;
// 初始化next数组,如果当前字符等于前一个字符,跳过;否则,寻找最长公共前缀
for (int i = 1, j = 0; i < len; ++i) {
while (j != -1 && pattern[i] != pattern[j + 1]) {
j = next[j]; // 如果当前字符不是之前的,从上次的失败位置开始查找
}
if (pattern[i] == pattern[j + 1]) { // 如果相等,说明找到了一个匹配
j++;
}
next[i] = j; // 将结果存入数组
}
return next;
}
// KMP 主函数
void kmpSearch(char* text, char* pattern, int* next) {
int textLen = strlen(text), patternLen = strlen(pattern);
int index = 0, matchIndex = 0;
while (index < textLen && matchIndex < patternLen) {
if (text[index] == pattern[matchIndex]) {
index++;
matchIndex++;
} else {
if (matchIndex != 0) {
matchIndex = next[matchIndex - 1]; // 从下一个next值处开始搜索
} else {
index++;
}
}
}
if (matchIndex == patternLen) {
printf("Pattern found at position %d\n", index - patternLen); // 打印匹配的位置
} else {
printf("Pattern not found in the text.\n");
}
}
int main() {
char t[] = "abcaabbabcab";
char p[] = "abca"; // 模式串
int *next = computeNext(p);
kmpSearch(t, p, next);
free(next); // 释放next数组内存
return 0;
}
```
在这个代码片段中,`computeNext` 函数用于计算模式串 `pattern` 的 next 数组,`kmpSearch` 函数则是实际的搜索过程。注意,在实际使用中,你需要将 `main` 中的模式串 `p` 替换为你想要查找的目标串。
阅读全文
相关推荐
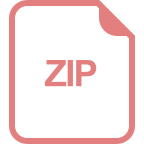
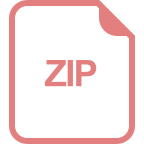
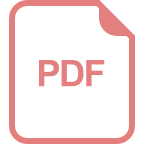



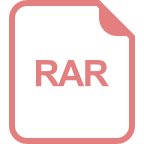
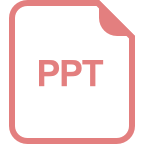
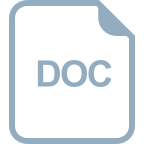
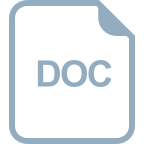
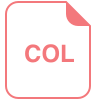
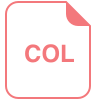
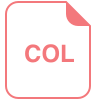
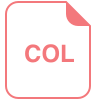
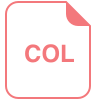


