c语言用空格拆分字符串,不使用strtok函数
时间: 2024-10-26 18:16:10 浏览: 25
在C语言中,如果不使用`strtok`函数,可以手动遍历字符串并逐个字符判断是否为空格,然后将非空格的部分存储到新数组或动态分配的内存里。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void split_string(const char *input, char **tokens, int *count) {
// 初始化状态变量
int i = 0;
*count = 0;
// 指针用于遍历输入字符串
const char *token_start = input;
while (true) {
// 找到下一个非空格字符
while (*token_start == ' ' || *token_start == '\t') {
token_start++;
if (*token_start == '\0') {
break; // 如果遇到字符串结束符,则停止
}
}
// 如果找到非空格字符则开始处理
if (*token_start != '\0') {
tokens[i] = (char *)malloc(strlen(token_start) + 1); // 分配空间
strcpy(tokens[i], token_start);
(*count)++;
// 移动指针到下一个空格位置或字符串结束
while (*token_start != ' ' && *token_start != '\t' && *token_start != '\0') {
token_start++;
}
} else {
break; // 当前字符串已经结束
}
// 如果还有剩余字符并且不是空格,则继续处理
i++;
}
// 结束标记
tokens[*count] = NULL;
}
int main() {
char str[] = "Hello World, This is a test string.";
char **result = malloc(sizeof(char*) * 10); // 预计最大10个单词
int count = 0;
split_string(str, result, &count);
for (int i = 0; i < count; i++) {
printf("%s\n", result[i]);
}
for (int i = 0; i < count; i++) {
free(result[i]); // 释放每个单词的内存
}
free(result); // 释放结果数组
return 0;
}
```
阅读全文
相关推荐
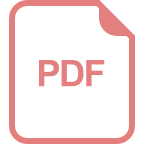
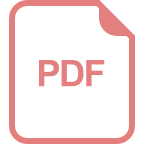
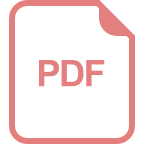




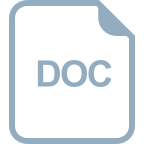










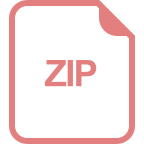