python利用xlrd遍历excel表,并利用pytest做数据驱动
时间: 2023-08-15 07:58:34 浏览: 112
要使用`xlrd`库遍历Excel表并使用`pytest`进行数据驱动测试,你可以按照以下步骤进行操作:
1. 首先,确保你已经安装了`xlrd`和`pytest`库。你可以使用以下命令通过pip安装它们:
```shell
pip install xlrd
pip install pytest
```
2. 创建一个Excel文件,其中包含你的测试数据。假设你的Excel文件名为`test_data.xlsx`,并且数据位于名为`Sheet1`的工作表中。
3. 创建一个名为`test_data.py`的Python文件,并在其中编写测试用例和测试数据。以下是一个示例:
```python
import xlrd
import pytest
# 读取Excel文件
def read_excel_file(file_path):
workbook = xlrd.open_workbook(file_path)
sheet = workbook.sheet_by_name('Sheet1')
data = []
for row in range(1, sheet.nrows):
test_case = {
'input': sheet.cell(row, 0).value,
'expected_output': sheet.cell(row, 1).value
}
data.append(test_case)
return data
# 测试用例
@pytest.mark.parametrize('test_data', read_excel_file('test_data.xlsx'))
def test_my_function(test_data):
input_data = test_data['input']
expected_output = test_data['expected_output']
# 执行测试逻辑,比如调用待测试的函数或方法
actual_output = my_function(input_data)
# 断言实际输出与期望输出是否一致
assert actual_output == expected_output
```
在上面的示例中,`read_excel_file`函数用于读取Excel文件并返回测试数据列表。`test_my_function`是一个测试用例函数,使用`@pytest.mark.parametrize`装饰器将测试数据作为参数传递给该函数。在测试用例函数中,你可以执行你的测试逻辑并使用断言来验证实际输出与期望输出是否一致。
请确保根据你的实际情况修改上述代码中的文件路径、工作表名称和列索引。
4. 运行测试。在命令行中进入包含`test_data.py`文件的目录,并运行以下命令:
```shell
pytest
```
`pytest`将自动运行测试用例,并根据测试结果提供相应的输出。
这样,你就可以使用`xlrd`库遍历Excel表并利用`pytest`进行数据驱动测试了。
阅读全文
相关推荐
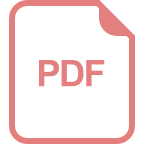
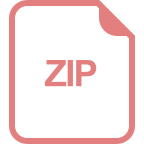
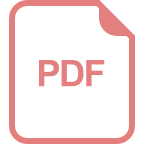















