python GRPC 服务端 收到 客户端的 消息后,关闭
时间: 2024-12-20 17:23:46 浏览: 6

基于gRPC实现的异步推理框架
在Python中,如果你正在使用gRPC(Google的远程过程调用协议)创建服务端,并希望在接收到客户端的消息后关闭服务,你需要在gRPC服务器的回调函数中添加适当的逻辑来控制服务的生命周期。gRPC提供了一个`TerminationInterceptor`,允许你在接收特定信号时关闭服务。
首先,确保你安装了gRPC库:
```bash
pip install grpcio protobuf
```
然后,在服务端实现中,你可以这样处理:
```python
from concurrent import futures
import grpc
import your_service_pb2_grpc as service_grpc
import your_service_pb2
class YourService(service_grpc.YourServiceServicer):
def YourMethod(self, request, context):
# 检查请求是否包含特殊标记或关闭信号
if should_shutdown(request): # 自定义逻辑判断条件
context.set_code(grpc.StatusCode.UNAVAILABLE)
context.set_details('Shutting down on client message.')
return your_service_pb2.Empty()
# 正常业务逻辑...
response = ... # 根据请求生成响应
return response
def should_shutdown(request):
# 判断逻辑,比如请求中携带了特殊的标识符或者满足其他条件
return request.some_flag or request.should_close
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10),
interceptors=(your_termination_interceptor,))
service_grpc.add_YourServiceServicer_to_server(YourService(), server)
server.add_insecure_port('[::]:50051') # gRPC监听地址
print("Starting server. Press Ctrl+C to stop.")
server.start()
try:
server.wait_for_termination() # 等待接收到客户端关闭信号或超时
except KeyboardInterrupt:
server.stop(0) # 用户手动中断时,优雅关闭
serve()
```
在这个例子中,`should_shutdown`函数可以根据客户端发送的消息内容检查是否需要关闭服务。如果需要,就调用`context.set_code`和`context.set_details`来告知客户端服务已关闭,然后返回一个空响应。最后,当`wait_for_termination`抛出`KeyboardInterrupt`异常时(通常是用户按下Ctrl+C),服务器会优雅地停止。
阅读全文
相关推荐
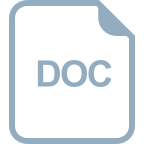
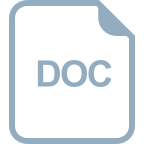


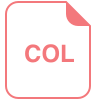
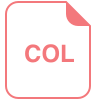
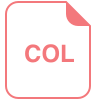
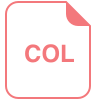
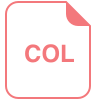
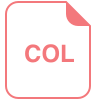

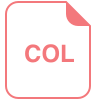
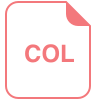




