C语言请定义一个整形数组,例如:ElemType data[9] = { 11,-22,33,-3,-88,21,77,0,-9 };将其插入顺序表,并在表中完成顺序表的基本操作(删除、增加、查找、取值等)。
时间: 2024-10-08 17:04:25 浏览: 44
在C语言中,你可以定义一个整型数组就像你给出的例子那样:
```c
typedef int ElemType; // 定义数据类型别名,方便操作
ElemType data[9] = { 11, -22, 33, -3, -88, 21, 77, 0, -9 };
```
接下来,我们可以创建一个顺序表结构体,包含一个指向数组元素的指针以及数组的长度:
```c
typedef struct {
ElemType* elements; // 数组元素的指针
int size; // 当前元素的数量
int capacity; // 序列的最大容量
} List;
```
为了将这个数组插入顺序表并完成基本操作,我们需要实现几个函数:
1. **初始化**:如果数组的大小小于最大容量,可以直接使用数组;否则复制到新的动态分配的空间。
```c
void initList(List* list, int capacity) {
list->elements = malloc(capacity * sizeof(ElemType));
list->size = 0;
list->capacity = capacity;
}
```
2. **插入**:找到合适的位置并将新元素添加到数组末尾。
```c
void insert(List* list, int index, const ElemType value) {
if (index < 0 || index > list->size) {
printf("Invalid index\n");
return;
}
if (list->size == list->capacity) {
resizeList(list);
}
memmove(&list->elements[index + 1], &list->elements[index], sizeof(ElemType) * (list->size - index));
list->elements[index] = value;
list->size++;
}
// 扩容函数,如果必要则分配更多内存
void resizeList(List* list) {
list->elements = realloc(list->elements, (list->capacity *= 2) * sizeof(ElemType));
}
```
3. **删除**:移除指定位置的元素。
```c
void delete(List* list, int index) {
if (index < 0 || index >= list->size) {
printf("Invalid index\n");
return;
}
memmove(&list->elements[index], &list->elements[index + 1], sizeof(ElemType) * (list->size - index - 1));
list->size--;
if (list->size < list->capacity / 4 && list->capacity != 0) {
resizeList(list); // 如果列表过小,考虑缩小容量
}
}
```
4. **查找/取值**:通过索引获取元素。
```c
ElemType search(List* list, int index) {
if (index < 0 || index >= list->size) {
printf("Invalid index\n");
return -1; // 返回某个特定标识符表示未找到
}
return list->elements[index];
}
```
5. **遍历**:显示整个序列。
```c
void display(List* list) {
for (int i = 0; i < list->size; i++) {
printf("%d ", list->elements[i]);
}
printf("\n");
}
```
阅读全文
相关推荐
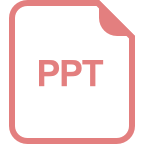
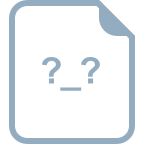
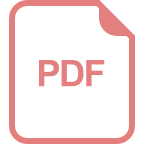















