请定义一个整形数组,例如1: ElemType data[9]=(11,-22,33,-3,-88,21,77,0,9};将 其插入顺序表,并在表中完成顺序表的基本操作(删除、增加、查找、取值等)。
时间: 2024-10-08 12:04:00 浏览: 63
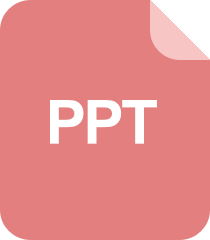
N维数组的顺序存储表示见教材P-C语言数据结构课件【比较清晰】
首先,我们定义一个整型结构体作为顺序表的节点,包含数据元素和指向下一个节点的指针:
```c++
typedef struct ListNode {
int data;
ListNode* next;
} ListNode;
```
然后,我们需要创建一个函数来初始化顺序表,即插入给定的数组:
```c++
void insertArray(ListNode** head, const int arr[], int size) {
if (*head == NULL) {
*head = new ListNode{arr[0], NULL};
}
for (int i = 1; i < size; ++i) {
ListNode* newNode = new ListNode{arr[i], *head};
*head = newNode;
}
}
```
对于顺序表的基本操作,我们可以分别编写如下函数:
1. **删除**:删除特定位置的数据,需要遍历找到目标节点并调整相邻节点的指针。
```c++
void deleteNode(ListNode** head, int index) {
if (*head == NULL) return;
if (index == 0) {
ListNode* temp = *head;
*head = (*head)->next;
delete temp;
} else {
ListNode* current = *head;
for (int i = 0; i < index - 1 && current != NULL; ++i) {
current = current->next;
}
if (current == NULL) return;
current->next = current->next->next;
delete current->next;
}
}
```
2. **增加**:在指定位置添加新元素,先找到该位置,然后插入新的节点。
```c++
void addNode(ListNode** head, int index, int value) {
if (index <= 0) return;
ListNode* newNode = new ListNode{value, NULL};
if (index == 1) {
newNode->next = *head;
*head = newNode;
} else {
ListNode* current = *head;
for (int i = 0; i < index - 1; ++i) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
```
3. **查找**:搜索特定值,在列表中返回第一个匹配的元素索引,如果没有找到则返回-1。
```c++
int search(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) return current - head;
current = current->next;
}
return -1;
}
```
4. **取值**:获取特定位置的数据,同样通过遍历来访问元素。
```c++
int getValue(ListNode* head, int index) {
if (index <= 0 || head == NULL) return -1;
ListNode* current = head;
for (int i = 0; i < index; ++i) {
current = current->next;
}
return current->data;
}
```
阅读全文
相关推荐
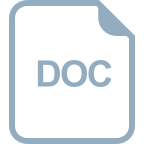
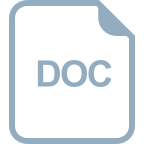















