内存错误bst* Deletebts(bst* t, Elemtype x) { bst* tmp; if (!t) printf("未找到待删除节点\n"); else if (!t->Lchild) { tmp = t->Rchild; free(t); return tmp; } else if (!t->Rchild) { tmp = t->Lchild; free(t); return tmp; } if (x < t->data) t->Lchild = Deletebts(t->Lchild, x); else if (x > t->data) t->Rchild = Deletebts(t->Rchild, x); else { if (t->Lchild && t->Rchild) { tmp = t->Rchild; while (tmp->Lchild) tmp = tmp->Lchild; t->data = tmp->data; t->Rchild = Deletebts(t->Rchild, t->data); } else { tmp = t; if (!t->Lchild) t = t->Rchild; else if (!t) t = t->Lchild; free(tmp); } } return t; }
时间: 2024-03-04 19:49:15 浏览: 62
代码中存在一个潜在的内存错误。当需要删除的节点只有左子树或右子树时,该节点被释放后,返回的是其子树的根节点,而没有将该节点的父节点的对应指针更新。这可能会导致在后续操作中,访问已经被释放的节点,从而导致内存错误。
修改方法是,在删除节点时,除了释放该节点的内存外,还需要更新其父节点的指针,使其指向该节点的子树的根节点。具体实现方式如下:
```c
bst* Deletebts(bst* t, Elemtype x) {
bst* tmp;
if (!t) {
printf("未找到待删除节点\n");
} else if (!t->Lchild) {
tmp = t->Rchild;
free(t);
t = tmp; // 更新父节点的指针
} else if (!t->Rchild) {
tmp = t->Lchild;
free(t);
t = tmp; // 更新父节点的指针
} else {
if (x < t->data) {
t->Lchild = Deletebts(t->Lchild, x);
} else if (x > t->data) {
t->Rchild = Deletebts(t->Rchild, x);
} else {
if (t->Lchild && t->Rchild) {
tmp = t->Rchild;
while (tmp->Lchild) {
tmp = tmp->Lchild;
}
t->data = tmp->data;
t->Rchild = Deletebts(t->Rchild, t->data);
} else {
tmp = t;
if (!t->Lchild) {
t = t->Rchild;
} else if (!t->Rchild) {
t = t->Lchild;
}
free(tmp);
}
}
}
return t;
}
```
在代码中,当节点只有左子树或右子树时,更新其父节点的指针,使其指向其子树的根节点。这样做可以避免在后续操作中访问已经被释放的节点,从而避免内存错误。
阅读全文
相关推荐
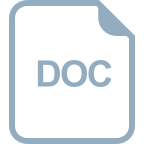
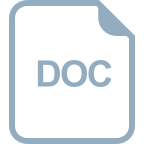
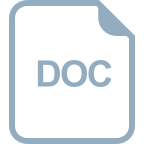





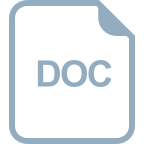
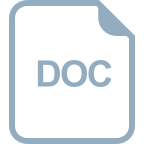
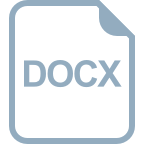
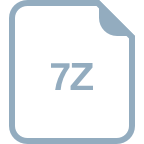
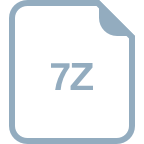
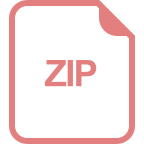
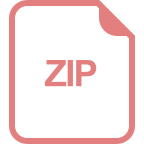