哈希函数的线性探测法Java实现
时间: 2024-04-16 17:27:12 浏览: 29
哈希函数的线性探测法是一种解决哈希冲突的方法,它通过在哈希表中寻找下一个可用的位置来存储冲突的元素。下面是一个简单的Java实现示例:
```java
public class LinearProbingHashTable {
private int capacity; // 哈希表容量
private int[] table; // 哈希表数组
public LinearProbingHashTable(int capacity) {
this.capacity = capacity;
this.table = new int[capacity];
}
// 哈希函数
private int hash(int key) {
return key % capacity;
}
// 插入元素
public void insert(int key) {
int index = hash(key);
// 线性探测找到下一个可用位置
while (table[index] != 0) {
index = (index + 1) % capacity;
}
// 插入元素
table[index] = key;
}
// 查找元素
public boolean search(int key) {
int index = hash(key);
// 线性探测查找元素
while (table[index] != 0) {
if (table[index] == key) {
return true;
}
index = (index + 1) % capacity;
}
return false;
}
// 删除元素
public void delete(int key) {
int index = hash(key);
// 线性探测查找并删除元素
while (table[index] != 0) {
if (table[index] == key) {
table[index] = -1; // 使用特殊标记表示已删除
return;
}
index = (index + 1) % capacity;
}
}
}
```
这是一个简单的线性探测法哈希表的Java实现。你可以根据需要修改和扩展这个实现。
相关推荐
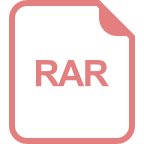
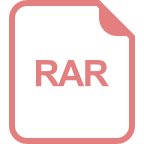
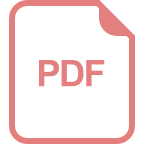














