直方图均衡化c语言代码
时间: 2024-11-05 15:08:37 浏览: 18
直方图均衡化是一种常见的图像处理技术,用于增强图像的对比度,使得图像中的细节更容易被观察到。在C语言中实现直方图均衡化的步骤通常包括计算图像的直方图、确定累积分布函数(CDF)、查找表(LUT),以及应用LUT到原始图像上。
以下是一个简单的C语言代码示例,它假设你已经有了一个灰度图像数组`image`和其宽度和高度(`width`, `height`):
```c
#include <stdio.h>
#include <stdlib.h>
// 定义LUT大小
#define TABLE_SIZE 256
// 创建直方图
int histogram[256] = {0};
void calculate_histogram(unsigned char* image, int width, int height) {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
histogram[image[x]]++;
}
}
}
// 计算累积分布函数
double cumulative_distribution(int value, int total_pixels) {
return ((double)value * (double)TABLE_SIZE / total_pixels);
}
// 根据CDF创建LUT
unsigned char* create_lookuptable() {
unsigned char lut[TABLE_SIZE] = {0};
double cdf = 0;
for (int i = 0; i < TABLE_SIZE; i++) {
if (histogram[i] != 0) {
cdf += histogram[i];
lut[i] = (unsigned char)(cdf * TABLE_SIZE); // 转换回像素值范围
} else {
lut[i] = lut[i - 1];
}
}
return lut;
}
// 应用LUT到图像上
void apply_lut(unsigned char* image, int width, int height, unsigned char* lut) {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
image[x] = lut[image[x]];
}
}
}
int main() {
// 具体的图像数据和尺寸...
calculate_histogram(image, width, height);
unsigned char* lut = create_lookuptable();
apply_lut(image, width, height, lut);
// ...其他后续操作,如保存或显示处理后的图像
return 0;
}
阅读全文
相关推荐
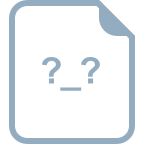
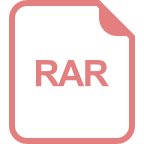
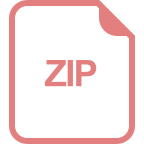














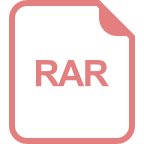
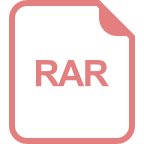