写代码① 对天气网进行分析;② 实现天气数据的爬取;③ 将获取的天气数据存储到MySQL中;④ 利用Sqoop将MySQL数据导入Hive。
时间: 2024-10-22 15:08:41 浏览: 39
编写这样的代码通常涉及以下几个步骤:
1. **天气数据分析**:首先,你需要使用网络爬虫库,如Python的`requests`和`BeautifulSoup`或`Scrapy`,从天气网站获取HTML数据。分析天气信息通常涉及到解析HTML结构,提取出温度、湿度、风速等关键天气参数。
```python
import requests
from bs4 import BeautifulSoup
url = "https://example.com/weather" # 替换为你想抓取的天气网站地址
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
weather_data = soup.find('div', class_='weather-data') # 假设这个div包含天气信息
```
2. **数据爬取**:对提取的数据进行清洗,并将其转化为可以处理的结构,比如字典或列表。然后根据需求,可能需要进行进一步的数据验证。
3. **存储到MySQL**:使用Python的数据库连接库,如`mysql-connector-python`,将数据插入MySQL数据库。设置好数据库连接并创建相应的表结构,如果表不存在则先创建。
```python
import mysql.connector
cnx = mysql.connector.connect(user='your_username', password='your_password',
host='localhost',
database='weather_db')
cursor = cnx.cursor()
query = ("INSERT INTO weather_table (temperature, humidity) VALUES (%s, %s)")
data = (weather_data['temperature'], weather_data['humidity']) # 假设这些键存在
cursor.execute(query, data)
cnx.commit()
cursor.close()
cnx.close()
```
4. **数据导入Hive**:使用Apache Sqoop工具,它允许你将数据从关系型数据库如MySQL迁移到Hadoop生态系统中的Hive。你需要配置Sqoop的环境变量,并运行命令行操作来执行迁移。
```sh
sqoop import --connect "jdbc:mysql://localhost/weather_db" \
--username your_username \
--password your_password \
--table weather_table \
--target-dir hdfs:///path/to/hive/warehouse/weather_table \
--hive-import
```
记得替换上述命令中的数据库连接信息、表名和目标目录。
阅读全文
相关推荐
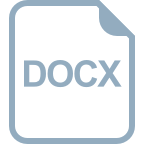
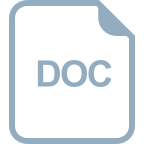
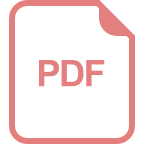
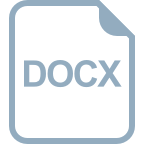
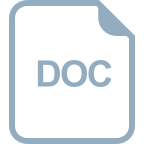
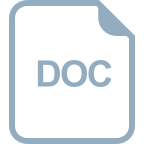
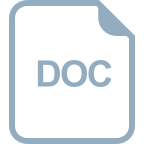
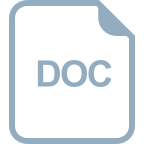
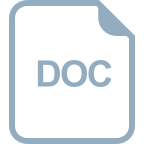
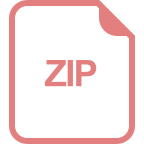
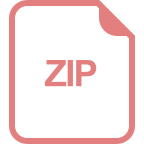
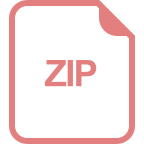
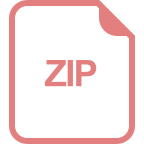