用C语言创建一个具有10个节点的单向链表,其数据域只有1个int的成员变量;给成员变量赋1-100间的随机值;然后,删除其中数值为奇数的节点;输出删除前后的链表内容
时间: 2024-04-14 10:29:25 浏览: 53
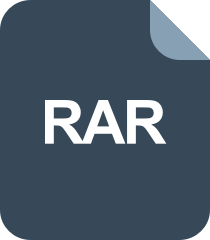
C语言实现单向链表的创建、插入,删除节点,和2个链表合并

好的,我将使用C语言为您创建一个具有10个节点的单向链表,并删除数值为奇数的节点。以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 链表节点结构
struct Node {
int data;
struct Node* next;
};
// 创建一个节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表末尾插入节点
void insertNode(struct Node** head, int data) {
struct Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
} else {
struct Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 删除数值为奇数的节点
void deleteOddNodes(struct Node** head) {
struct Node* current = *head;
struct Node* prev = NULL;
while (current != NULL) {
if (current->data % 2 != 0) {
if (prev == NULL) {
*head = current->next;
free(current);
current = *head;
} else {
prev->next = current->next;
free(current);
current = prev->next;
}
} else {
prev = current;
current = current->next;
}
}
}
// 打印链表内容
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
srand(time(NULL));
struct Node* head = NULL;
for (int i = 0; i < 10; i++) {
int randomValue = rand() % 100 + 1;
insertNode(&head, randomValue);
}
printf("删除前的链表内容:");
printList(head);
deleteOddNodes(&head);
printf("删除后的链表内容:");
printList(head);
return 0;
}
```
该代码会先创建一个具有10个节点的单向链表,并给每个节点的数据域赋予1-100间的随机值。然后,它会删除数值为奇数的节点,并输出删除前后的链表内容。
请注意,由于使用了随机数生成器,每次运行结果可能会有所不同。
阅读全文
相关推荐
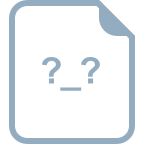
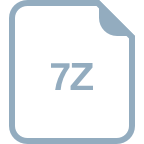
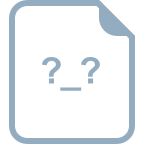
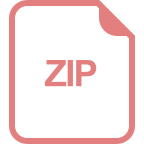
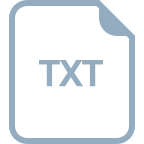
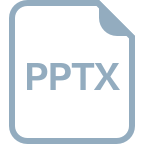
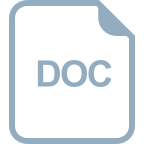
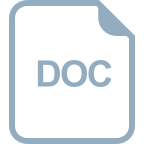
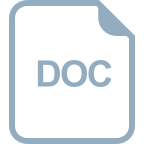
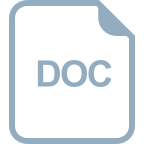
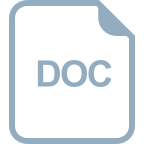
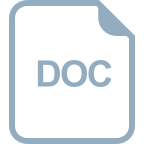
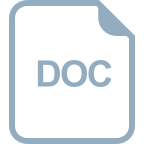
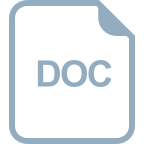
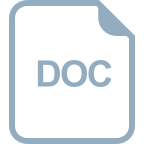
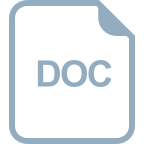
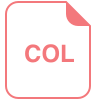