for k in kernels: TypeError: 'int' object is not iterable
时间: 2023-11-12 10:59:41 浏览: 154
这个错误通常是因为你将一个整数对象传递给了需要迭代的函数或方法。例如,在这个错误中,`kernels` 可能是一个整数,而你尝试在 `for` 循环中迭代它。请确保 `kernels` 是一个可迭代的对象,例如列表或元组。
如果 `kernels` 是一个整数,你可以将其转换为一个包含该整数的列表,然后再进行迭代。例如:
```
kernels = 3
for k in [kernels]:
# do something with k
```
如果 `kernels` 是一个列表或元组,那么请检查它是否被正确地定义和初始化。
相关问题
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement the mean-shift algorithm :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the mean-shift algorithm return
Sure! Here's one possible implementation of the mean-shift algorithm:
```python
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""
Implement the mean-shift algorithm
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return:
the estimated means with size (N, D)
"""
N, D = xs.shape
means = xs.copy()
for i in range(num_iter):
for j, x in enumerate(xs):
# compute the kernel weights
if k_type == 'rbf':
w = np.exp(-0.5 * np.sum((x - means) ** 2, axis=1) / bandwidth ** 2)
elif k_type == 'gate':
w = np.exp(-np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'triangle':
w = np.maximum(0, 1 - np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'linear':
w = np.sum((x - means) * xs, axis=1) / (np.linalg.norm(x - means, axis=1) * np.linalg.norm(x))
else:
raise ValueError("Unsupported kernel type: {}".format(k_type))
# update the mean by weighted average
means[j] = np.sum(w[:, np.newaxis] * xs, axis=0) / np.sum(w)
return means
```
In this implementation, we start with the initial means being the same as the input samples, and then iteratively update the means by computing the kernel weights between each sample and the current means, and then computing the weighted average of the samples using these weights. The kernel can be selected using the `k_type` parameter, and the kernel width can be controlled by the `bandwidth` parameter. The number of iterations can be controlled by the `num_iter` parameter. Finally, the estimated means are returned.
Continue to refine the following code and don't add any other packages but numpy: def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement a variant of mean-shift algorithm, with unchanged kernel matrix :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the modified mean-shift return xs
Here is a possible implementation of the modified mean-shift algorithm:
```
def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""Implement a variant of mean-shift algorithm, with unchanged kernel matrix
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return: the estimated means with size (N, D)
"""
N, D = xs.shape
# Initialize the means as the input samples
ms = xs.copy()
# Define the kernel function
if k_type == 'rbf':
k = lambda x, y: np.exp(-0.5 * np.linalg.norm(x - y)**2 / bandwidth**2)
elif k_type == 'gate':
k = lambda x, y: np.exp(-0.5 * np.abs(x - y) / bandwidth)
elif k_type == 'triangle':
k = lambda x, y: np.maximum(0, 1 - np.abs(x - y) / bandwidth)
elif k_type == 'linear':
k = lambda x, y: np.dot(x, y)
else:
raise ValueError('Unknown kernel type')
# Iterate to find the means
for _ in range(num_iter):
# Compute the kernel matrix
K = np.zeros((N, N))
for i in range(N):
for j in range(i, N):
K[i, j] = K[j, i] = k(xs[i], xs[j])
# Compute the weights and the updated means
weights = K.sum(axis=1)
ms_new = np.zeros((N, D))
for i in range(N):
ms_new[i] = (K[i, :, np.newaxis] * xs).sum(axis=0) / weights[i]
# Check for convergence
if np.allclose(ms, ms_new, rtol=1e-5):
break
ms = ms_new
return ms
```
In this implementation, we first initialize the means as the input samples. Then we define the kernel function based on the input `k_type` and `bandwidth`. We use a nested loop to compute the kernel matrix, which has size (N, N). We then use the kernel matrix to compute the weights and the updated means for each sample. We repeat this process for `num_iter` iterations or until the means converge. Finally, we return the estimated means. Note that this implementation only uses numpy and does not rely on any other packages.
阅读全文
相关推荐
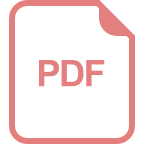
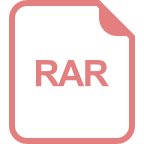
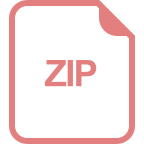
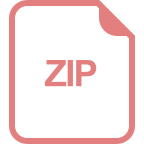
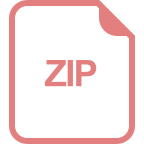
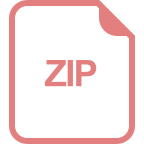
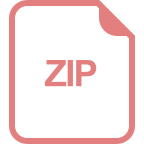
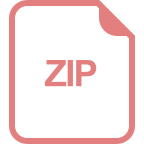
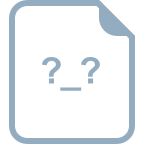
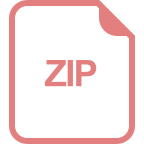
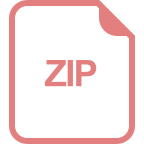
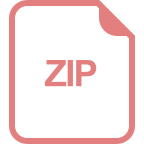
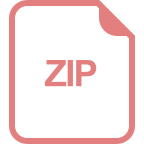
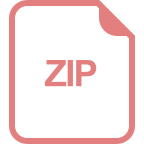


