c++ map判断是否key
时间: 2023-11-12 07:08:22 浏览: 202
在C++中,可以使用count函数或find函数来判断map中是否存在某个key值。count函数用于统计key值在map中出现的次数,如果key存在返回1,不存在返回0。而find函数则返回一个迭代器,如果找到了对应的key,则指向该key所对应的键值对,否则指向map的尾部。下面是使用count函数和find函数判断map中是否存在某个key值的示例代码:
```
// 假设已经定义了一个名为testMap的map对象
int key = 1;
if (testMap.count(key) == 0) {
cout << "no this key" << endl;
} else {
cout << "key exists" << endl;
}
// 或者使用find函数
auto iter = testMap.find(key);
if (iter == testMap.end()) {
cout << "no this key" << endl;
} else {
cout << "key exists, value is " << iter->second << endl;
}
```
相关问题
c++ map判断是否存在
在C++中,`std::map` 是一种关联容器,用于存储键值对。如果你想判断一个键是否已经存在于 `std::map` 中,你可以使用 `find` 函数。以下是一个示例:
```cpp
#include <map>
#include <string>
std::map<std::string, int> myMap; // 假设这是一个已初始化的 map
// 判断键 "key" 是否存在
bool exists = myMap.find("key") != myMap.end();
if (exists) {
std::cout << "键 'key' 已经存在。\n";
} else {
std::cout << "键 'key' 不存在。\n";
}
```
`find(key)` 会查找指定的键,如果找到则返回该键对应的迭代器,否则返回 `myMap.end()`。所以,如果 `find(key)` 返回的迭代器不等于 `myMap.end()`,那就说明键存在。
c++ map string做key
是一个常见的做法。在 C++ 中,`std::map` 是一个关联容器,它存储着一些 key-value 对,其中 key 按照一定的顺序进行排序,且每个 key 唯一。因此,如果你需要使用字符串作为 key,可以使用 `std::map<std::string, ...>` 来定义该容器。例如:
```c++
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> myMap;
// 插入 key-value 对
myMap["hello"] = 1;
myMap["world"] = 2;
// 访问 key 对应的 value
std::cout << myMap["hello"] << std::endl; // 输出 1
std::cout << myMap["world"] << std::endl; // 输出 2
return 0;
}
```
在上面的例子中,我们定义了一个 `std::map<std::string, int>` 类型的容器 `myMap`,并插入了两个 key-value 对。然后,我们使用 `myMap["hello"]` 和 `myMap["world"]` 来访问相应的 value 值。由于 key 是字符串类型的,因此我们需要用双引号将其括起来。
阅读全文
相关推荐
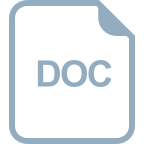
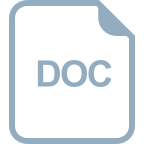
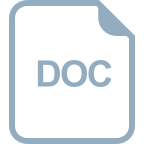













