c++ map 获取所有key
时间: 2023-09-06 09:14:15 浏览: 247
可以通过遍历 map 中的所有元素,获取所有的 key。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "one"},
{2, "two"},
{3, "three"},
{4, "four"}
};
// 遍历 map 获取所有 key
std::cout << "All keys in the map: ";
for (auto const& element : myMap) {
std::cout << element.first << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:
```
All keys in the map: 1 2 3 4
```
在上面的代码中,我们使用了 C++11 的 range-based for 循环来遍历 map 中的所有元素,然后通过 `element.first` 获取每个元素的 key。
相关问题
c++ map 获取key列表
在 C++ 中,Map 是一个关联数组,它将键映射到值。如果你想要获取 Map 中所有的键列表,可以使用迭代器。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
#include <vector>
int main() {
std::map<int, std::string> my_map{{1, "one"}, {2, "two"}, {3, "three"}};
std::vector<int> keys;
for (auto const& element : my_map) {
keys.push_back(element.first);
}
for (auto const& key : keys) {
std::cout << key << " ";
}
return 0;
}
```
在上面的代码中,我们首先声明了一个 Map 对象 my_map,并向其中添加了三个键值对。接下来,我们声明一个 vector 对象 keys,用于存储 Map 中的所有键。然后,我们使用一个 for 循环来遍历 Map 中的所有元素,将每个键添加到 keys 向量中。最后,我们再次使用 for 循环来遍历 keys 向量,并输出每个键。
运行上面的代码,输出将是:
```
1 2 3
```
这就是 Map 中所有的键列表。
c++ map获取key值
你可以使用C++ STL中的`map`容器来获取key值。`map`是一个关联式容器,它将键映射到值。你可以使用`map`的`find()`函数来查找一个键,并返回一个迭代器,该迭代器指向包含该键的元素。然后你可以使用迭代器的`->first`来访问该元素的键,即key值。以下是一个示例代码:
```cpp
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> m;
m["apple"] = 1;
m["banana"] = 2;
m["cherry"] = 3;
//查找"banana"的位置
auto it = m.find("banana");
if (it != m.end()) {
cout << "key: " << it->first << ", value: " << it->second << endl;
}
else {
cout << "not found" << endl;
}
return 0;
}
```
输出结果为:
```
key: banana, value: 2
```
相关推荐
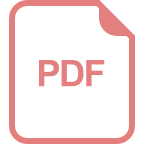
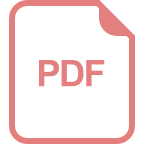
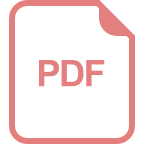












