c++打印map中的所有key
时间: 2024-01-04 21:46:35 浏览: 49
在 C 语言中,我们可以使用 `map` 类型的变量来存储一组键值对,其中每个键都是唯一的,并且可以通过键来访问对应的值。要打印 `map` 中的所有键,我们可以遍历整个 `map`,并打印每个键。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include <map>
int main() {
std::map<int, char*> my_map;
my_map[1] = "apple";
my_map[2] = "banana";
my_map[3] = "cherry";
// 遍历 map 并打印所有键
std::map<int, char*>::iterator it;
for(it = my_map.begin(); it != my_map.end(); it++) {
int key = it->first;
printf("%d\n", key);
}
return 0;
}
```
在上面的代码中,我们首先定义了一个名为 `my_map` 的 `map` 变量,并将三个键值对插入到 `map` 中。然后,我们使用迭代器 `it` 遍历整个 `map`,并使用 `it->first` 来访问每个键,并打印它们。输出结果为:
```
1
2
3
```
相关问题
c++ map 获取所有key
可以通过遍历 map 中的所有元素,获取所有的 key。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "one"},
{2, "two"},
{3, "three"},
{4, "four"}
};
// 遍历 map 获取所有 key
std::cout << "All keys in the map: ";
for (auto const& element : myMap) {
std::cout << element.first << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:
```
All keys in the map: 1 2 3 4
```
在上面的代码中,我们使用了 C++11 的 range-based for 循环来遍历 map 中的所有元素,然后通过 `element.first` 获取每个元素的 key。
怎么取出C++中 map 的key
在 C 语言中,没有内置的 Map(映射)类型。但是,你可以使用结构体来模拟 Map。
假设你有一个结构体 `map_node`,包含 `key` 和 `value` 两个字段:
```c
struct map_node {
char *key;
int value;
};
```
你可以创建一个数组 `map` 来保存这些结构体。然后,你可以使用循环遍历数组,取出每个结构体的 `key` 字段,如下所示:
```c
for (int i = 0; i < map_size; i++) {
char *key = map[i].key;
printf("Key: %s\n", key);
}
```
注意,这里假设 `map_size` 是数组的大小。你可以根据实际情况修改这个值。另外,如果你要在 Map 中存储字符串,需要使用动态内存分配来分配内存。同时,你需要记得在使用完字符串后释放它们的内存,以避免内存泄漏。
相关推荐
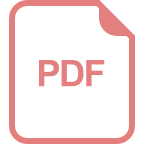
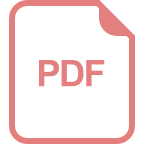
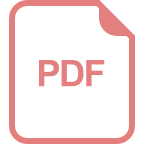












