float ControlComply::BiaDisLimitSpeed(const float tBiaDistance) { float distance_array[5] = {0}; float speed_array[5] = {0}; ros::param::get("lateral_distance0", distance_array[0]); ros::param::get("lateral_distance1", distance_array[1]); ros::param::get("lateral_distance2", distance_array[2]); ros::param::get("lateral_distance3", distance_array[3]); ros::param::get("lateral_distance4", distance_array[4]); ros::param::get("speed_dis_limit0", speed_array[0]); ros::param::get("speed_dis_limit1", speed_array[1]); ros::param::get("speed_dis_limit2", speed_array[2]); ros::param::get("speed_dis_limit3", speed_array[3]); ros::param::get("speed_dis_limit4", speed_array[4]); return pubalgor.FuzzyDataProcess(distance_array, speed_array, 5, tBiaDistance); }
时间: 2024-04-17 18:27:22 浏览: 24
这段代码定义了一个名为`BiaDisLimitSpeed`的函数,该函数接受一个`float`类型的参数`tBiaDistance`。
在函数内部,定义了两个数组变量`distance_array`和`speed_array`,它们分别包含了5个元素。这些数组将用于存储从ROS参数服务器中获取的横向距离和速度限制数据。
接下来,使用`ros::param::get`方法从ROS参数服务器中获取具体的参数值,并将它们存储在相应的数组元素中。例如,`ros::param::get("lateral_distance0", distance_array[0])`表示从ROS参数服务器中获取名为`lateral_distance0`的参数值,并将其存储在数组`distance_array`的第一个元素`distance_array[0]`中。
最后,调用了`pubalgor.FuzzyDataProcess(distance_array, speed_array, 5, tBiaDistance)`方法,并将获取到的横向距离和速度限制数据作为参数传递给该方法。该方法可能是对这些数据进行模糊处理,并返回一个结果值。
函数最终将这个结果值作为函数的返回值。
总结来说,这段代码定义了一个函数,用于从ROS参数服务器中获取横向距离和速度限制数据,并对这些数据进行模糊处理并返回结果。
相关问题
bool IsGreenLight(uint8_t light_state); //speed limit float CurveLimitSpeed(const float tCurve); float BiaAngleLimitSpeed(const float tBiaAngle); float BiaDisLimitSpeed(const float tBiaDistance); float SpeedJudge(float tDesireSpeed);//m/s
你供的代码段是一组函数声明,它们分别为IsGreenLight、CurveLimitSpeed、BiaAngleLimitSpeed、BiaDisLimitSpeed和SpeedJudge。
IsGreenLight函数接受一个uint8_t类型的参数light_state,并返回一个bool类型的值。根据函数名和参数名字来看,这个函数可能用于判断给定的交通灯状态是否为绿灯,并返回相应的布尔值。
CurveLimitSpeed函数接受一个float类型的参数tCurve,并返回一个float类型的值。根据函数名和参数名字来看,这个函数可能是用于根据给定的曲率限制车辆的速度,并返回相应的限制速度。
BiaAngleLimitSpeed函数接受一个float类型的参数tBiaAngle,并返回一个float类型的值。根据函数名和参数名字来看,这个函数可能是用于根据给定的偏航角限制车辆的速度,并返回相应的限制速度。
BiaDisLimitSpeed函数接受一个float类型的参数tBiaDistance,并返回一个float类型的值。根据函数名和参数名字来看,这个函数可能是用于根据给定的偏航距离限制车辆的速度,并返回相应的限制速度。
SpeedJudge函数接受一个float类型的参数tDesireSpeed,并返回一个float类型的值。根据函数名和参数名字来看,这个函数可能是用于根据给定的期望速度进行速度判断或计算,并返回相应的速度。
然而,从你提供的代码中无法得知这些函数的具体实现细节。如果你需要进一步了解这些函数的实现或者有其他相关问题,请提供更多的代码上下文或者描述具体的需求,我将尽力提供帮助。
优化extern const float segmentTheta = 60.0/180.0*M_PI;
可以使用宏定义来优化这行代码,将60.0和180.0使用宏定义,如下所示:
```
#define SEGMENT_THETA_DEG 60.0
#define SEGMENT_THETA_RAD (SEGMENT_THETA_DEG / 180.0 * M_PI)
extern const float segmentTheta = SEGMENT_THETA_RAD;
```
这样做的好处是,如果需要修改角度值,只需要修改宏定义即可,而不需要在多个地方手动修改数值。同时,使用宏定义可以让代码更加易读,更容易理解。
相关推荐
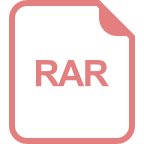
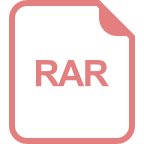
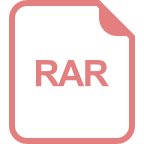












