#include<iostream> using namespace std; class Array{ // 数组类 public: Array(float *p,int m,float x1); // 用p初始化a,m初始化n,x1初始化x ~Array(); // 释放动态空间 void print()const; // 按指定格式输出结果 void count(); // 计算比x大的元素个数 private: float *a,x; // 数组及指定的x int n; // 数组大小(所有元素个数) static int number; // 数组中比x大的元素个数 }; //**********found********** __________________________ ; Array::Array(float *p,int m,float x1):n(m),x(x1) { int i; //**********found********** __________________________ ; for(i=0;i<n;i++) a[i]=p[i]; } Array::~Array() { //**********found********** __________________________ ; } void Array::print()const { int i; cout<<"数组为:\n"; for(i=0;i<n;i++) cout<<a[i]<<'\t'; cout<<endl; cout<<"x为:"<<x<<endl; cout<<"比"<<x<<"大的元素个数为:"<<number<<endl; } void Array::count() { int i; for(i=0;i<n;i++) //**********found********** _________________ number++; } int main() { float t[9]={6,8,3,9,4,2,1,7,5}; Array test(t,9,5.0); test.count(); test.print(); system("pause"); return 0; }
时间: 2023-07-23 17:12:20 浏览: 99
在代码中缺少了两个函数的实现,需要填充代码:
```c++
#include<iostream>
using namespace std;
class Array{ // 数组类
public:
Array(float *p,int m,float x1); // 用p初始化a,m初始化n,x1初始化x
~Array(); // 释放动态空间
void print()const; // 按指定格式输出结果
void count(); // 计算比x大的元素个数
private:
float *a,x; // 数组及指定的x
int n; // 数组大小(所有元素个数)
static int number; // 数组中比x大的元素个数
};
int Array::number = 0;
Array::Array(float *p,int m,float x1):n(m),x(x1) {
int i;
a = new float[n];
for(i=0;i<n;i++)
a[i]=p[i];
}
Array::~Array() {
delete[] a;
}
void Array::print()const {
int i;
cout<<"数组为:\n";
for(i=0;i<n;i++)
cout<<a[i]<<'\t';
cout<<endl;
cout<<"x为:"<<x<<endl;
cout<<"比"<<x<<"大的元素个数为:"<<number<<endl;
}
void Array::count() {
int i;
for(i=0;i<n;i++)
if(a[i] > x)
number++;
}
int main() {
float t[9]={6,8,3,9,4,2,1,7,5};
Array test(t,9,5.0);
test.count();
test.print();
system("pause");
return 0;
}
```
这是一个比较简单的数组类实现,类中包含了构造函数、析构函数、输出函数和计算比x大的元素个数的函数。在主函数中,我们创建了一个数组对象,并调用count函数计算比x大的元素个数,最后输出结果。
阅读全文
相关推荐
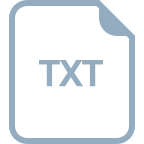
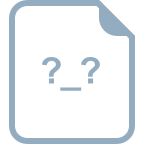















