modbus_new_tcp
时间: 2023-08-02 21:02:05 浏览: 97
modbus_new_tcp是一个用于建立Modbus TCP连接的函数。Modbus是一种常用的工业通信协议,主要用于在工业自动化系统中进行设备间的通信。而Modbus TCP是基于TCP/IP协议的一种实现方式。
modbus_new_tcp函数的作用是创建一个Modbus TCP连接,并返回一个用于操作该连接的句柄。在使用该函数之前,需要事先准备好正确的IP地址和端口号,以便与Modbus设备建立连接。
使用modbus_new_tcp函数可以方便地与Modbus设备进行通信。首先,使用该函数创建一个Modbus TCP连接句柄,然后通过句柄进行读写操作。可以使用modbus_read_registers函数来读取Modbus设备的寄存器数据,使用modbus_write_registers函数来写入数据到Modbus设备的寄存器。
需要注意的是,在使用完毕后,需要通过modbus_close函数关闭Modbus TCP连接,并通过modbus_free函数释放相关资源,防止资源泄漏。同时,关闭连接后,连接句柄将不再可用,需要重新使用modbus_new_tcp函数创建新的连接。
总之,modbus_new_tcp是一个用于建立Modbus TCP连接的函数,可以方便地与Modbus设备进行通信。通过该函数,我们可以读取和写入Modbus设备的数据,实现设备间的数据交互。
相关问题
libmodbus的modbus_new_tcp和modbus_new_tcp_pi的区别
在libmodbus库中,modbus_new_tcp()和modbus_new_tcp_pi()是用于创建Modbus TCP连接的函数,它们有一些区别。
1. modbus_new_tcp():
- 这个函数用于创建简单的Modbus TCP连接。
- 它接受IP地址和端口号作为参数。
- 它返回一个指向Modbus上下文的指针,如果出现错误,则返回NULL。
- 一旦成功创建连接,就可以使用其他的modbus函数来进行读写操作。
2. modbus_new_tcp_pi():
- 这个函数用于创建带有握手过程的Modbus TCP连接。
- 它接受IP地址和端口号作为参数。
- 它返回一个指向Modbus上下文的指针,如果出现错误,则返回NULL。
- 与modbus_new_tcp()不同,它会在握手过程中使用TCP/IP协议来建立连接。
- 这种握手过程提供了更高的安全性和可靠性,但可能会对性能产生一些影响。
总结来说,modbus_new_tcp()和modbus_new_tcp_pi()函数都可以用于创建Modbus TCP连接,但前者是简单的连接方式,而后者是带有握手过程的连接方式。选择哪个函数取决于您的需求,如果对安全性和可靠性要求较高,可以选择modbus_new_tcp_pi(),否则可以选择modbus_new_tcp()。
#include <iostream> #include "libmodbus/modbus.h" #include <stdexcept> class ModbusTCPServer { public: ModbusTCPServer(int port) { ctx = modbus_new_tcp(NULL, port); if (!ctx) { throw std::runtime_error("Failed to create Modbus TCP server."); } } ~ModbusTCPServer() { modbus_close(ctx); modbus_free(ctx); } void listen(int max_connections) { modbus_tcp_listen(ctx, max_connections); } void accept(modbus_t* slave_ctx) { modbus_tcp_accept(ctx, &slave_ctx); } void close() { modbus_tcp_close(ctx); } private: modbus_t* ctx; };错误
这段代码中存在一个错误:在`accept`函数中,`&slave_ctx`应该改为`slave_ctx`,即:
```
void accept(modbus_t* slave_ctx) {
modbus_tcp_accept(ctx, slave_ctx);
}
```
因为`modbus_tcp_accept`函数的第二个参数是一个`modbus_t**`类型的指针,而`slave_ctx`已经是一个指向`modbus_t`类型的指针,不需要再取其地址。
另外,建议在`listen`函数和`accept`函数中添加错误检查,以确保这些函数执行成功。
修改后的代码如下:
```
#include <iostream>
#include "libmodbus/modbus.h"
#include <stdexcept>
class ModbusTCPServer {
public:
ModbusTCPServer(int port) {
ctx = modbus_new_tcp(NULL, port);
if (!ctx) {
throw std::runtime_error("Failed to create Modbus TCP server.");
}
}
~ModbusTCPServer() {
modbus_close(ctx);
modbus_free(ctx);
}
void listen(int max_connections) {
if (modbus_tcp_listen(ctx, max_connections) == -1) {
throw std::runtime_error("Failed to listen for Modbus TCP connections.");
}
}
void accept(modbus_t* slave_ctx) {
if (modbus_tcp_accept(ctx, slave_ctx) == -1) {
throw std::runtime_error("Failed to accept Modbus TCP connection.");
}
}
void close() {
modbus_tcp_close(ctx);
}
private:
modbus_t* ctx;
};
```
这样就能保证代码能够正确地运行,并在发生错误时抛出异常。
相关推荐
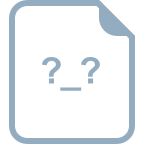












