实现简单的对称加密用于设备和服务器之间的通信,安全通信的实现c++语言 引入加密库或者编写简单的对称加密函数 对socket传输的所有敏感数据进行加密处理 分别提供设备端和服务端代码
时间: 2024-10-14 20:16:03 浏览: 18
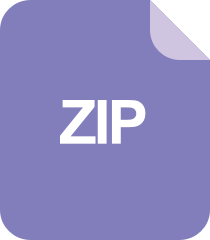
基于QT实现的RAS非对称加密的信息传输系统c++源码+项目说明.zip
在C++中,我们可以使用像`Crypto++`这样的开源加密库来进行对称加密,它提供了丰富的加密算法如AES(Advanced Encryption Standard)。为了实现设备和服务器之间的安全通信,我们通常会选择一种常见的对称加密模式,如AES加解密操作。
首先,你需要安装`Crypto++`库,如果还没有安装,可以访问https://www.cryptopp.com/ 下载并按照指示配置。
**设备端(客户端)代码示例:**
```cpp
#include <cryptopp/aes.h>
#include <iostream>
#include <string>
#include <boost/asio.hpp>
std::string encrypt(const std::string &plaintext, const std::string &key) {
CryptoPP::AES::Encryption aesEncryption(key);
CryptoPP::AutoSeededRandomPool prng;
CryptoPP:: BufferedTransformation encryptionTransform(aesEncryption, prng);
CryptoPP::StringSource plaintextSource(plaintext, true,
new CryptoPP::StreamTransformationFilter(encryptionTransform,
new CryptoPP::StringSink()));
return encryptionTransform.GetLastOutput();
}
std::string decrypt(const std::string &ciphertext, const std::string &key) {
CryptoPP::AES::Decryption aesDecryption(key);
// 同样的随机数生成器和缓冲流处理
// 只需要将aesEncryption换成aesDecryption即可
// ...
}
int main() {
boost::asio::io_context ioContext;
std::string data = "Sensitive Data";
std::string key = "YourSecretKey"; // 使用的加密密钥
auto encryptedData = encrypt(data, key);
// 使用Socket发送加密后的数据...
// 接收服务端发来的数据,并解密
std::string receivedEncryptedData = ...; // 从网络接收
auto decryptedData = decrypt(receivedEncryptedData, key);
std::cout << "Decrypted Data: " << decryptedData << std::endl;
return 0;
}
```
**服务端(服务器端)代码示例:**
```cpp
#include <cryptopp/aes.h>
#include <iostream>
#include <string>
#include <boost/asio.hpp>
// 服务端的加密、解密函数与客户端类似,只需将接收到的数据传递给decrypt函数,然后返回给客户端
int main() {
boost::asio::io_context ioContext;
std::string receivedEncryptedData = ...; // 从网络接收的数据
std::string key = "YourSecretKey"; // 同样使用共享的加密密钥
std::string decryptedData = decrypt(receivedEncryptedData, key);
// 使用Socket发送解密后的数据...
// 接收客户端请求,对其发送数据进行加密...
...
return 0;
}
```
阅读全文
相关推荐
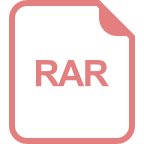
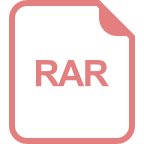

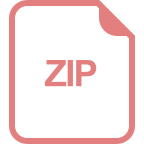
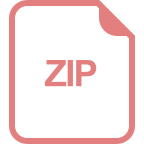
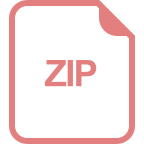
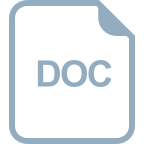
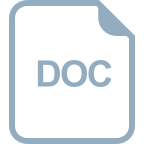
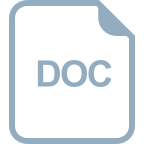
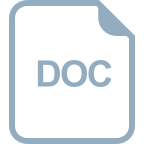
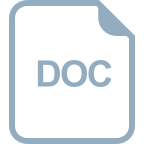
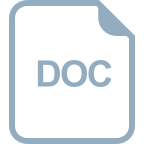
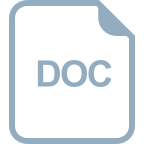
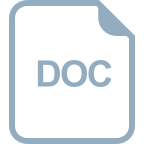

