本题要求实现一个函数,遍历一个不带头节点的链表,求链表节点数据的最大值 节点类型定义: struct node { int ch ; struct node *next ;} 函数接口定义: 在这里描述函数接口。例如: int max_node( struct node *p) p是链表头指针,返回链表上最大的ch属性值。 裁判测试程序样例: #include <stdio.h> #include<stdlib.h> struct node {int ch; struct node * next;}; struct node *setlink(int N);//建立链表函数,已经定义 int max_node(struct node * head);//需要定义这个函数 int main() { int N; struct node *head; scanf("%d",&N); head=setlink(N); printf("%d", max_node(head)); return 0; } 输入样例: 在这里给出一组输入。例如: 6 7 8 9 1 2 3 输出样例: 在这里给出相应的输出。例如: 9
时间: 2024-04-13 11:30:49 浏览: 157
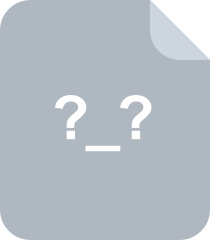
单链表的接口函数实现以及相关接口函数的测试
你可以使用以下代码实现这个函数:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int ch;
struct node *next;
};
int max_node(struct node *head) {
if (head == NULL) { // 如果链表为空,返回0
return 0;
}
int max = head->ch; // 初始化最大值为第一个节点的值
struct node *current = head->next;
while (current != NULL) {
if (current->ch > max) { // 如果当前节点的值比最大值大,更新最大值
max = current->ch;
}
current = current->next; // 移动到下一个节点
}
return max;
}
// 测试代码
struct node *setlink(int N) {
struct node *head = NULL;
struct node *prev = NULL;
for (int i = 0; i < N; i++) {
struct node *newNode = (struct node *)malloc(sizeof(struct node));
scanf("%d", &(newNode->ch));
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
prev->next = newNode;
}
prev = newNode;
}
return head;
}
int main() {
int N;
struct node *head;
scanf("%d", &N);
head = setlink(N);
printf("%d", max_node(head));
return 0;
}
```
这段代码首先定义了一个节点结构体 `node`,包含一个整数属性 `ch` 和一个指向下一个节点的指针 `next`。
然后是 `max_node` 函数,它接收一个链表的头指针 `head`,并返回链表节点数据的最大值。函数首先判断链表是否为空,如果为空则返回0。然后使用一个变量 `max` 来记录当前最大值,初始化为第一个节点的值。然后使用一个循环遍历链表的每个节点,如果当前节点的值比 `max` 大,则更新 `max` 的值。最后返回 `max`。
为了测试这个函数,提供了一个 `setlink` 函数来构建链表。它接收一个整数 `N`,表示链表的长度,然后从输入中读取 `N` 个整数,并构建相应的链表。最后在 `main` 函数中调用 `setlink` 函数来创建链表,并调用 `max_node` 函数来求解最大值,并输出结果。
阅读全文
相关推荐
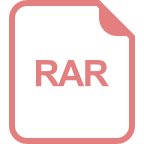




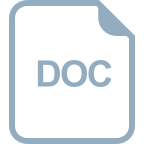








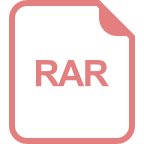