can’t write to interface object “y” of mode IN 这个问题怎么解决
时间: 2024-10-13 13:04:26 浏览: 17
这个错误提示"can't write to interface object "y" of mode IN"通常出现在编程中,特别是处理文件输入流(InputStream)或者数据接口的时候。当尝试向一个只读(IN)的对象写入数据时,就会发生这种错误。
解决这个问题的关键在于理解对象的访问模式(mode)。在这个例子中,你需要确认“y”对象是否确实是一个可以写入的数据源,而不是一个只读的输入流。以下是几个可能的解决方案:
1. **检查对象初始化**:确保你在创建“y”对象时正确设置了它的模式为可写(如FileOutputStream或BufferedWriter),而不是默认的只读。
```java
FileOutputStream fos = new FileOutputStream("file.txt", true); // 指定追加写入模式
```
2. **更改写操作**:如果你试图写入的是一个接口对象,确保你不是在一个预期只能读取的方法里试图写入内容。检查并确保调用的是允许写入的方法。
3. **检查权限**:如果是在操作系统层面的限制,确保有足够的权限去写入指定的文件或路径。
4. **异常处理**:在代码中添加适当的异常处理,捕获`IOException`并在其中处理可能出现的写入权限不足的情况。
如果以上步骤都无法解决问题,可能是其他程序层面的问题,比如并发访问、锁控制等。查看相关的代码段以及日志信息会更有帮助。
相关问题
Modify the getfile.py used during class to allow user to enter a customized file name instead of fixed original file name. The interface should have three data controls: (1) The file Selector, which can be made by using “input name=file1 type=file ” (2) The saving file name, which can be made by using “input name=fn ” (3) The Submit button, which can be made by “input type=submit name=submit value=Upload ” Don't use template. Submit the python program
Sure, here's the modified `getfile.py` script that allows the user to enter a customized file name:
```python
#!/usr/bin/env python3
import cgi
import os
# Set the upload directory
UPLOAD_DIR = '/path/to/upload/directory/'
# Create the form object
form = cgi.FieldStorage()
# Get the uploaded file object
fileitem = form['file1']
# Get the custom file name from the form
filename = form['fn'].value
# Check if the file was uploaded
if fileitem.filename:
# Create the upload directory if it doesn't exist
if not os.path.isdir(UPLOAD_DIR):
os.makedirs(UPLOAD_DIR)
# Set the file path
filepath = UPLOAD_DIR + filename
# Open the file for writing in binary mode
with open(filepath, 'wb') as f:
# Read the file in chunks
while True:
chunk = fileitem.file.read(1024)
if not chunk:
break
# Write the chunk to the file
f.write(chunk)
# Print a success message
print('Content-type:text/html\r\n\r\n')
print('<html>')
print('<head>')
print('<title>File Upload</title>')
print('</head>')
print('<body>')
print('<h2>File Uploaded Successfully</h2>')
print('</body>')
print('</html>')
else:
print('Content-type:text/html\r\n\r\n')
print('<html>')
print('<head>')
print('<title>File Upload Error</title>')
print('</head>')
print('<body>')
print('<h2>Error: No File Specified</h2>')
print('</body>')
print('</html>')
```
This script uses the `cgi` module to handle the form data, and allows the user to enter a custom file name using an additional form input. The custom file name is then used to save the uploaded file to the upload directory.
用springboot+vue写一个前后分离的登录功能
好的,下面是一个简单的示例:
1. 后端使用Spring Boot搭建,创建一个名为"login"的Spring Boot项目。
2. 在项目中添加依赖:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL Driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- JSON Web Token -->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
</dependencies>
```
3. 创建一个名为"User"的实体类,用于表示用户信息。
```java
@Entity
@Table(name = "users")
public class User implements UserDetails {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
@Column(nullable = false)
private String role;
// getters and setters
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return Arrays.asList(new SimpleGrantedAuthority(role));
}
@Override
public boolean isAccountNonExpired() {
return true;
}
@Override
public boolean isAccountNonLocked() {
return true;
}
@Override
public boolean isCredentialsNonExpired() {
return true;
}
@Override
public boolean isEnabled() {
return true;
}
}
```
4. 创建一个名为"UserRepository"的接口,用于操作用户数据。
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
5. 创建一个名为"JwtUtils"的工具类,用于生成和验证JSON Web Token。
```java
public class JwtUtils {
private static final String SECRET_KEY = "secret";
public static String generateToken(UserDetails userDetails) {
Map<String, Object> claims = new HashMap<>();
claims.put("username", userDetails.getUsername());
claims.put("role", userDetails.getAuthorities().stream().findFirst().get().getAuthority());
return Jwts.builder()
.setClaims(claims)
.setSubject(userDetails.getUsername())
.setExpiration(new Date(System.currentTimeMillis() + 60 * 60 * 1000))
.signWith(SignatureAlgorithm.HS512, SECRET_KEY)
.compact();
}
public static boolean validateToken(String token, UserDetails userDetails) {
String username = getUsernameFromToken(token);
return username.equals(userDetails.getUsername()) && !isTokenExpired(token);
}
public static String getUsernameFromToken(String token) {
return Jwts.parser().setSigningKey(SECRET_KEY).parseClaimsJws(token).getBody().getSubject();
}
public static boolean isTokenExpired(String token) {
return Jwts.parser().setSigningKey(SECRET_KEY).parseClaimsJws(token).getBody().getExpiration().before(new Date());
}
}
```
6. 创建一个名为"JwtAuthenticationFilter"的过滤器,用于处理登录请求并生成JSON Web Token。
```java
public class JwtAuthenticationFilter extends UsernamePasswordAuthenticationFilter {
private final AuthenticationManager authenticationManager;
public JwtAuthenticationFilter(AuthenticationManager authenticationManager) {
this.authenticationManager = authenticationManager;
setFilterProcessesUrl("/api/login");
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) throws AuthenticationException {
try {
User user = new ObjectMapper().readValue(request.getInputStream(), User.class);
return authenticationManager.authenticate(new UsernamePasswordAuthenticationToken(user.getUsername(), user.getPassword(), Collections.emptyList()));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response, FilterChain chain, Authentication authResult) throws IOException, ServletException {
String token = JwtUtils.generateToken((UserDetails) authResult.getPrincipal());
response.setContentType("application/json");
response.getWriter().write("{\"token\": \"" + token + "\"}");
}
}
```
7. 创建一个名为"SecurityConfig"的配置类,用于配置Spring Security。
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserRepository userRepository;
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(username -> userRepository.findByUsername(username)).passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/login").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JwtAuthenticationFilter(authenticationManager()))
.addFilter(new JwtAuthorizationFilter(authenticationManager()))
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
}
```
8. 创建一个名为"JwtAuthorizationFilter"的过滤器,用于验证请求中的JSON Web Token。
```java
public class JwtAuthorizationFilter extends BasicAuthenticationFilter {
private static final String HEADER_STRING = "Authorization";
private static final String TOKEN_PREFIX = "Bearer ";
public JwtAuthorizationFilter(AuthenticationManager authenticationManager) {
super(authenticationManager);
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException {
String header = request.getHeader(HEADER_STRING);
if (header != null && header.startsWith(TOKEN_PREFIX)) {
String token = header.substring(TOKEN_PREFIX.length());
try {
String username = JwtUtils.getUsernameFromToken(token);
UserDetails userDetails = userDetailsService().loadUserByUsername(username);
if (JwtUtils.validateToken(token, userDetails)) {
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
SecurityContextHolder.getContext().setAuthentication(authentication);
}
} catch (JwtException e) {
SecurityContextHolder.clearContext();
}
}
chain.doFilter(request, response);
}
}
```
9. 前端使用Vue.js搭建,创建一个名为"login"的Vue.js项目。
10. 在项目中安装axios和vue-router:
```
npm install --save axios vue-router
```
11. 创建一个名为"Login"的组件,用于渲染登录表单。
```vue
<template>
<div>
<h1>Login</h1>
<form @submit.prevent="login">
<div>
<label for="username">Username:</label>
<input type="text" id="username" v-model="username">
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" v-model="password">
</div>
<div>
<button type="submit">Login</button>
</div>
</form>
</div>
</template>
<script>
import axios from 'axios'
export default {
data () {
return {
username: '',
password: ''
}
},
methods: {
login () {
axios.post('/api/login', { username: this.username, password: this.password })
.then(response => {
localStorage.setItem('token', response.data.token)
this.$router.push('/')
})
.catch(error => {
console.error(error)
})
}
}
}
</script>
```
12. 创建一个名为"App"的组件,用于渲染主页面。
```vue
<template>
<div>
<h1>Home</h1>
<div v-if="authenticated">
<p>Welcome, {{ username }}!</p>
<button @click="logout">Logout</button>
</div>
<div v-else>
<router-link to="/login">Login</router-link>
</div>
</div>
</template>
<script>
import axios from 'axios'
export default {
data () {
return {
authenticated: false,
username: ''
}
},
created () {
axios.get('/api/user')
.then(response => {
this.authenticated = true
this.username = response.data.username
})
.catch(error => {
console.error(error)
})
},
methods: {
logout () {
localStorage.removeItem('token')
this.$router.push('/')
location.reload()
}
}
}
</script>
```
13. 创建一个名为"router"的路由配置文件,用于配置Vue.js路由。
```js
import Vue from 'vue'
import VueRouter from 'vue-router'
import Login from './components/Login.vue'
import App from './components/App.vue'
Vue.use(VueRouter)
const router = new VueRouter({
mode: 'history',
routes: [
{ path: '/', component: App },
{ path: '/login', component: Login }
]
})
router.beforeEach((to, from, next) => {
const token = localStorage.getItem('token')
if (to.path !== '/login' && !token) {
next('/login')
} else {
next()
}
})
export default router
```
14. 在"index.html"中添加Vue.js和axios的CDN链接:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
</head>
<body>
<div id="app"></div>
<script src="dist/build.js"></script>
</body>
</html>
```
15. 在"main.js"中创建Vue.js实例并挂载到DOM上:
```js
import Vue from 'vue'
import App from './components/App.vue'
import router from './router'
new Vue({
el: '#app',
router,
render: h => h(App)
})
```
16. 运行项目:
```
mvn spring-boot:run
```
```
npm run dev
```
现在你可以在浏览器中访问"http://localhost:8080"来测试登录功能了。
阅读全文
相关推荐
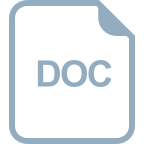
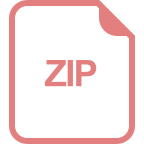
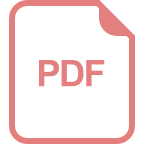
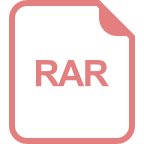
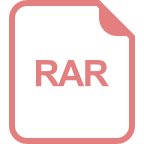
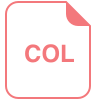
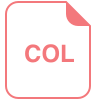
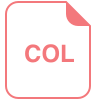
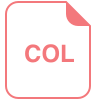
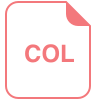
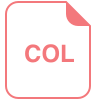
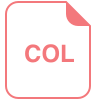
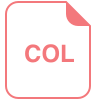
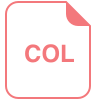
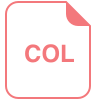
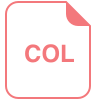
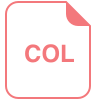