java StringRedisTemplate
时间: 2024-01-18 08:37:09 浏览: 70
Java StringRedisTemplate是Spring Data Redis库中的一个类,它用于与Redis数据库进行交互并操作存储在其中的字符串数据。
StringRedisTemplate提供了一系列方法来执行各种Redis操作,例如设置、获取、删除和更新键值对、对字符串进行追加、获取子字符串等。它还支持事务操作和管道操作,可以执行多个操作并将它们打包成一个原子性操作。
使用StringRedisTemplate,你可以方便地将字符串数据存储到Redis中,并可以通过键来检索、更新或删除这些数据。同时,它还提供了一些额外的功能,例如计数器和分布式锁,在处理分布式应用程序时非常有用。
需要注意的是,使用StringRedisTemplate之前,你需要在Spring配置文件中配置Redis连接信息,并将StringRedisTemplate注入到你的应用程序中以便使用。
相关问题
java stringredistemplate的使用
StringRedisTemplate是Spring Data Redis提供的用于操作Redis的模板类。它可以直接操作Redis中的String类型数据,使用起来非常方便。下面是StringRedisTemplate的使用示例:
1. 引入依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置Redis连接信息
在application.properties文件中添加以下配置信息:
```
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=
```
3. 定义StringRedisTemplate
在Java代码中定义StringRedisTemplate:
```
@Autowired
private StringRedisTemplate stringRedisTemplate;
```
4. 使用StringRedisTemplate操作数据
StringRedisTemplate提供了一系列操作Redis的方法,如set、get、incr等。以下是一些常用的示例代码:
```
// 设置key-value
stringRedisTemplate.opsForValue().set("name", "Tom");
// 获取value
String name = stringRedisTemplate.opsForValue().get("name");
// 自增1
stringRedisTemplate.opsForValue().increment("count");
// 批量设置key-value
Map<String, String> map = new HashMap<>();
map.put("name", "Tom");
map.put("age", "18");
stringRedisTemplate.opsForValue().multiSet(map);
// 批量获取value
List<String> values = stringRedisTemplate.opsForValue().multiGet(Arrays.asList("name", "age"));
```
以上就是StringRedisTemplate的使用示例。在实际开发中,我们可以根据需求灵活使用StringRedisTemplate提供的操作方法来操作Redis。
java StringRedisTemplate 给不同的database赋值
`StringRedisTemplate` 是 Spring Data Redis 的一部分,它提供了操作 Redis 的常用方法。在 Redis 中,通过不同的 database 可以实现数据的隔离存储。默认情况下,Redis 服务器启动时会创建 16 个数据库(database 0 到 database 15),每个数据库都是独立的命名空间,使用不同的数据库可以避免键名冲突。
使用 `StringRedisTemplate` 给不同数据库赋值的步骤如下:
1. 首先,获取 `RedisTemplate` 实例的引用。
2. 使用 `select(int dbIndex)` 方法选择特定的数据库。其中 `dbIndex` 是目标数据库的索引,从 0 开始到 15 结束。
3. 使用 `StringRedisTemplate` 提供的 `opsForValue()`、`opsForList()`、`opsForSet()` 等方法进行数据操作。
以下是一个简单的示例代码:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.stereotype.Component;
@Component
public class RedisExample {
@Autowired
private StringRedisTemplate stringRedisTemplate;
public void setValuesToDifferentDatabases() {
// 选择第一个数据库进行操作
stringRedisTemplate.setConnectionFactory(connectionFactory);
stringRedisTemplate.select(0); // 选择数据库0
stringRedisTemplate.opsForValue().set("key1", "value1_in_db0");
// 选择第二个数据库进行操作
stringRedisTemplate.select(1); // 选择数据库1
stringRedisTemplate.opsForValue().set("key2", "value2_in_db1");
// ...重复上述步骤,为其他数据库设置数据
}
}
```
注意:在实际应用中,不建议频繁切换数据库,因为每次切换数据库都会产生网络开销,影响性能。通常情况下,一次操作就选择一个数据库进行到底。
阅读全文
相关推荐
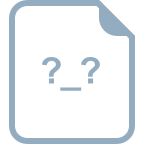













