Uncaught (in promise) TypeError: Cannot read properties of undefined (reading 'value')
时间: 2024-09-18 12:17:16 浏览: 159
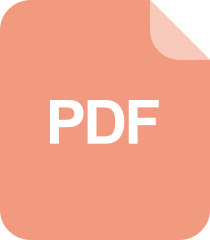
Vue报错:Uncaught TypeError: Cannot assign to read only property’exports‘ of object’#Object‘的解决方法
Uncaught (in promise) TypeError: Cannot read property 'value' of undefined 这是一个JavaScript错误,通常发生在尝试访问一个Promise对象的状态(通常是resolve()或reject()方法返回的值),但它还没有被解析(resolve)或拒绝(reject)。当你试图通过`value`属性获取这个状态时,由于Promise尚处于pending(等待中)状态,它没有对应的值,因此会抛出TypeError。
例如:
```javascript
const promise = new Promise((resolve, reject) => {
// 没有立即调用 resolve 或 reject
});
promise.value; // 抛出错误,因为 value 属性不存在,且 promise 未完成
```
为了解决这个问题,你需要确保你在Promise状态明确(已成功或失败)后再访问其结果,可以使用`.then()`和`.catch()`来处理:
```javascript
promise.then(value => {
console.log(value); // 在这里,如果Promise解析,你可以安全地读取 value
}).catch(error => {
console.error(error); // 如果Promise被拒绝,捕获并处理错误
});
```
阅读全文
相关推荐
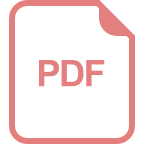
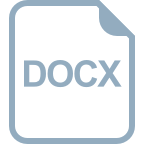
















